As you've discovered, both Quadratic curves and Cubic Bezier curves just connect 2 points with a curve.
Since the Cubic curve has more control points, it is more flexible in the path it takes between those 2 points.
For example, let’s say you want to draw this letter “R”:
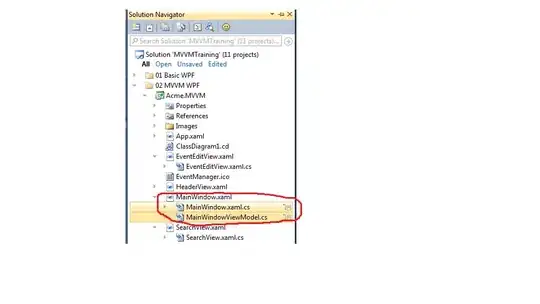
Start drawing with the “non-curvey” parts of the R:
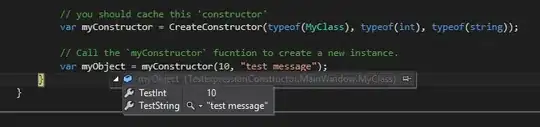
Now try drawing the curve with a quadratic curve.
Notice the quadratic curve is more “pointy” than what we desire.
That’s because we only have 1 control point to define quadratic curviness.
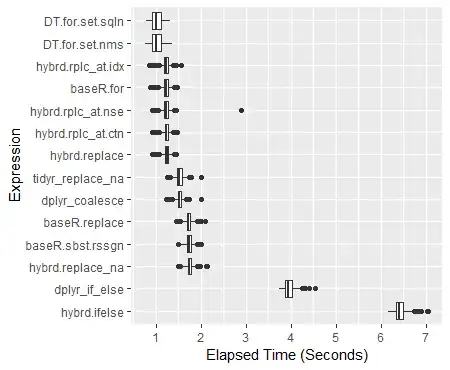
Now try drawing the curve with a cubic bezier curve.
The cubic bezier curve is more nicely rounded than the quadratic curve.
That’s because we have 2 control points to define cubic curviness.
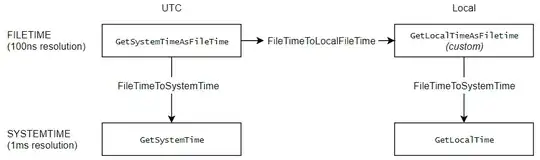
So...more control points gives more control over "curviness"
Here is code and a Fiddle: http://jsfiddle.net/m1erickson/JpXZW/
<!doctype html>
<html>
<head>
<link rel="stylesheet" type="text/css" media="all" href="css/reset.css" /> <!-- reset css -->
<script type="text/javascript" src="http://code.jquery.com/jquery.min.js"></script>
<style>
body{ background-color: ivory; padding:20px; }
#canvas{border:1px solid red;}
</style>
<script>
$(function(){
var canvas=document.getElementById("canvas");
var ctx=canvas.getContext("2d");
ctx.lineWidth=8;
ctx.lineCap="round";
function baseR(){
ctx.clearRect(0,0,canvas.width,canvas.height);
ctx.beginPath();
ctx.moveTo(30,200);
ctx.lineTo(30,50);
ctx.lineTo(65,50);
ctx.moveTo(30,120);
ctx.lineTo(65,120);
ctx.lineTo(100,200);
ctx.strokeStyle="black";
ctx.stroke()
}
function quadR(){
ctx.beginPath();
ctx.moveTo(65,50);
ctx.quadraticCurveTo(130,85,65,120);
ctx.strokeStyle="red";
ctx.stroke();
}
function cubicR(){
ctx.beginPath();
ctx.moveTo(65,50);
ctx.bezierCurveTo(120,50,120,120,65,120);
ctx.strokeStyle="red";
ctx.stroke();
}
$("#quad").click(function(){
baseR();
quadR();
//cubicR();
});
$("#cubic").click(function(){
baseR();
cubicR();
});
}); // end $(function(){});
</script>
</head>
<body>
<button id="quad">Use Quadratic curve</button>
<button id="cubic">Use Cubic Bezier curve</button><br><br>
<canvas id="canvas" width=150 height=225></canvas>
</body>
</html>