With the reshape2
(for reshaping your data into long format) and ggplot2
(for plotting) packages, it will be quite a lot easier to make such a plot.
The code:
dat <- read.table("http://dpaste.com/1563769/plain/",header=TRUE)
library(reshape2)
library(ggplot2)
# reshape your data into long format
long <- melt(dat, id=c("Method","Metric"),
measure=c("E0","E1","E2","E4"),
variable = "E.nr")
# make the plot
ggplot(long) +
geom_bar(aes(x = Method, y = value, fill = Metric),
stat="identity", position = "dodge", width = 0.7) +
facet_wrap(~E.nr) +
scale_fill_manual("Metric\n", values = c("red","blue"),
labels = c(" Precision", " Recall")) +
labs(x="",y="") +
theme_bw() +
theme(
panel.grid.major.y = element_line(colour = "black", linetype = 3, size = .5),
panel.background = element_blank(),
axis.title.x = element_text(size=16),
axis.text.x = element_text(size=14, angle=45, hjust=1, vjust=1),
axis.title.y = element_text(size=16, angle = 90),
axis.text.y = element_text(size=14),
strip.background = element_rect(color="white", fill="white"),
strip.text = element_text(size=16)
)
The result:
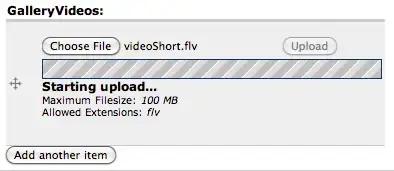
When you want to keep axis-labels on each seperate plot, you'll need the ggplot2
and gridExtra
packages.
The code:
dat <- read.table("http://dpaste.com/1563769/plain/",header=TRUE)
library(ggplot2)
library(gridExtra)
# making the seperate plots
pE0 <- ggplot(dat) +
geom_bar(aes(x = Method, y = E0, fill = Metric),
stat="identity", position = "dodge", width = 0.7) +
scale_fill_manual("Metric\n", values = c("red","blue"),
labels = c(" Precision", " Recall")) +
labs(title="E0\n",x="",y="") +
theme_bw() +
theme(
panel.grid.major.y = element_line(colour = "black", linetype = 3, size = .5),
panel.background = element_blank(),
axis.title.x = element_text(size=16),
axis.text.x = element_text(size=14, angle=30, hjust=1, vjust=1),
axis.title.y = element_text(size=16, angle = 90),
axis.text.y = element_text(size=14)
)
pE1 <- ggplot(dat) +
geom_bar(aes(x = Method, y = E1, fill = Metric),
stat="identity", position = "dodge", width = 0.7) +
scale_fill_manual("Metric\n", values = c("red","blue"),
labels = c(" Precision", " Recall")) +
labs(title="E1\n",x="",y="") +
theme_bw() +
theme(
panel.grid.major.y = element_line(colour = "black", linetype = 3, size = .5),
panel.background = element_blank(),
axis.title.x = element_text(size=16),
axis.text.x = element_text(size=14, angle=30, hjust=1, vjust=1),
axis.title.y = element_text(size=16, angle = 90),
axis.text.y = element_text(size=14)
)
pE2 <- ggplot(dat) +
geom_bar(aes(x = Method, y = E2, fill = Metric),
stat="identity", position = "dodge", width = 0.7) +
scale_fill_manual("Metric\n", values = c("red","blue"),
labels = c(" Precision", " Recall")) +
labs(title="E2\n",x="",y="") +
theme_bw() +
theme(
panel.grid.major.y = element_line(colour = "black", linetype = 3, size = .5),
panel.background = element_blank(),
axis.title.x = element_text(size=16),
axis.text.x = element_text(size=14, angle=30, hjust=1, vjust=1),
axis.title.y = element_text(size=16, angle = 90),
axis.text.y = element_text(size=14)
)
pE4 <- ggplot(dat) +
geom_bar(aes(x = Method, y = E4, fill = Metric),
stat="identity", position = "dodge", width = 0.7) +
scale_fill_manual("Metric\n", values = c("red","blue"),
labels = c(" Precision", " Recall")) +
labs(title="E4\n",x="",y="") +
theme_bw() +
theme(
panel.grid.major.y = element_line(colour = "black", linetype = 3, size = .5),
panel.background = element_blank(),
axis.title.x = element_text(size=16),
axis.text.x = element_text(size=14, angle=30, hjust=1, vjust=1),
axis.title.y = element_text(size=16, angle = 90),
axis.text.y = element_text(size=14)
)
# function to extract the legend (borrowed from: https://github.com/hadley/ggplot2/wiki/Share-a-legend-between-two-ggplot2-graphs )
g_legend<-function(a.gplot){
tmp <- ggplot_gtable(ggplot_build(a.gplot))
leg <- which(sapply(tmp$grobs, function(x) x$name) == "guide-box")
legend <- tmp$grobs[[leg]]
return(legend)}
legend <- g_legend(pE1)
lwidth <- sum(legend$width)
# combining the plots with gridExtra
grid.arrange(arrangeGrob(pE0 + theme(legend.position="none"),
pE1 + theme(legend.position="none"),
pE2 + theme(legend.position="none"),
pE4 + theme(legend.position="none")
),
legend, widths=unit.c(unit(1, "npc") - lwidth, lwidth), nrow=1)
The result:
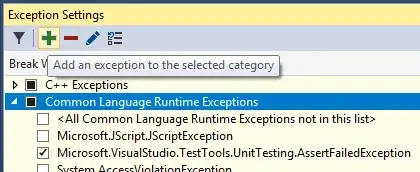