Why don't you just setLocationRelativeTo(parent)
instead of trying to set the location manually.
Side Notes:
Use Java naming convention. variable names start with lowecase letters. I see you have Parent
and parent
. That's where this
comes in handy
this.parent = parent
instead of
Parent = parent
It looks like you're trying to use two JFrame
. Why? There other more user friendly options like a model JDialog
or a CardLayout
. See The Use of Multiple JFrames, Good/Bad Practice?
UPDATE
Though I'm against the use of multiple frames, I will still give you an answer, Only because I took my time to actually test this out. Here are the steps I took in GUI Builder, which it looks like that's what you're using.
- I made two
JFrame
forms FrameOne
and FrameTwo
.
- I changed the
defaultCloseOperation
of FrameOne
to DISPOSE
- In
FrameTwo
I deleted the main
method because the application is already running from the FrameOne
class. I kept the look and feel code though. Just cop and pasted it to the constructor.
I Did this, set the location relative to the first frame
FrameOne frameOne;
public FrameTwo(FrameOne frameOne) {
initComponents();
this.frameOne = frameOne;
// look and feel code here
setLocationRelativeTo(frameOne);
setVisible(true);
}
Added a WindowListener
to the FrameOne
public FrameOne() {
initComponents();
addWindowListener(new WindowAdapter(){
@Override
public void windowClosing(WindowEvent e) {
new FrameTwo(FrameOne.this);
FrameOne.this.dispose();
}
});
}
It works fine.
FrameOne.java
UPATE with code from GU Builder
I added no components to FrameOne, just close the frame. The only things I did was what I mentioned in the steps above
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.GroupLayout;
import javax.swing.WindowConstants;
public class FrameOne extends javax.swing.JFrame {
public FrameOne() {
initComponents();
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
new FrameTwo(FrameOne.this);
FrameOne.this.dispose();
}
});
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
setDefaultCloseOperation(WindowConstants.DISPOSE_ON_CLOSE);
GroupLayout layout = new GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(GroupLayout.Alignment.LEADING)
.addGap(0, 400, Short.MAX_VALUE)
);
layout.setVerticalGroup(
layout.createParallelGroup(GroupLayout.Alignment.LEADING)
.addGap(0, 300, Short.MAX_VALUE)
);
pack();
}// </editor-fold>
public static void main(String args[]) {
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(FrameOne.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(FrameOne.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(FrameOne.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(FrameOne.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
//</editor-fold>
/* Create and display the form */
java.awt.EventQueue.invokeLater(new Runnable() {
public void run() {
new FrameOne().setVisible(true);
}
});
}
// Variables declaration - do not modify
// End of variables declaration
}
FrameTwo.java
import java.awt.Font;
import javax.swing.GroupLayout;
import javax.swing.JLabel;
import javax.swing.SwingConstants;
import javax.swing.WindowConstants;
public class FrameTwo extends javax.swing.JFrame {
private FrameOne frameOne;
public FrameTwo(final FrameOne frameOne) {
initComponents();
this.frameOne = frameOne;
setLocationRelativeTo(frameOne);
try {
for (javax.swing.UIManager.LookAndFeelInfo info : javax.swing.UIManager.getInstalledLookAndFeels()) {
if ("Nimbus".equals(info.getName())) {
javax.swing.UIManager.setLookAndFeel(info.getClassName());
break;
}
}
} catch (ClassNotFoundException ex) {
java.util.logging.Logger.getLogger(FrameTwo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (InstantiationException ex) {
java.util.logging.Logger.getLogger(FrameTwo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (IllegalAccessException ex) {
java.util.logging.Logger.getLogger(FrameTwo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
} catch (javax.swing.UnsupportedLookAndFeelException ex) {
java.util.logging.Logger.getLogger(FrameTwo.class.getName()).log(java.util.logging.Level.SEVERE, null, ex);
}
setVisible(true);
}
@SuppressWarnings("unchecked")
// <editor-fold defaultstate="collapsed" desc="Generated Code">
private void initComponents() {
jLabel1 = new JLabel();
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
jLabel1.setFont(new Font("Impact", 0, 36)); // NOI18N
jLabel1.setHorizontalAlignment(SwingConstants.CENTER);
jLabel1.setText("frame two");
GroupLayout layout = new GroupLayout(getContentPane());
getContentPane().setLayout(layout);
layout.setHorizontalGroup(
layout.createParallelGroup(GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(118, 118, 118)
.addComponent(jLabel1, GroupLayout.PREFERRED_SIZE, 161, GroupLayout.PREFERRED_SIZE)
.addContainerGap(121, Short.MAX_VALUE))
);
layout.setVerticalGroup(
layout.createParallelGroup(GroupLayout.Alignment.LEADING)
.addGroup(layout.createSequentialGroup()
.addGap(90, 90, 90)
.addComponent(jLabel1, GroupLayout.PREFERRED_SIZE, 89, GroupLayout.PREFERRED_SIZE)
.addContainerGap(121, Short.MAX_VALUE))
);
pack();
}// </editor-fold>
/* Set the Nimbus look and feel */
//<editor-fold defaultstate="collapsed" desc=" Look and feel setting code (optional) ">
/* If Nimbus (introduced in Java SE 6) is not available, stay with the default look and feel.
* For details see http://download.oracle.com/javase/tutorial/uiswing/lookandfeel/plaf.html
*/
//</editor-fold>
// Variables declaration - do not modify
private JLabel jLabel1;
// End of variables declaration
}
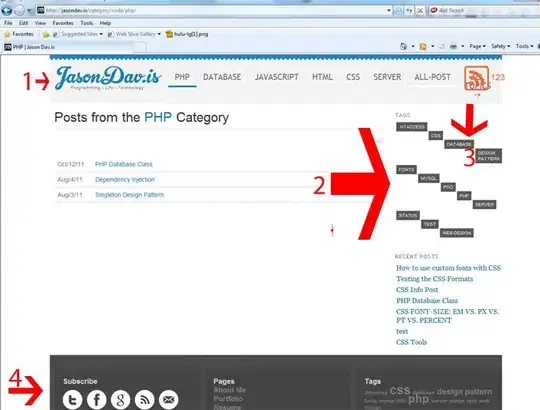