Basically, Frame 1
will need a reference to Frame 2
either directly or indirectly via some kind of observer implementation...
This way, all you need to do is provide some kind of method in Frame 2
that will allow you to pass the information you need to it so it can update the text area in question.
Personally, I prefer to use interface
s for this, as it prevents the caller from messing with things it shouldn't
Oh, and you might also want to have a read through The Use of Multiple JFrames: Good or Bad Practice?
For example...
The NotePad
interface
prevents SecretaryPane
from making changes to the underlying implementation, because the only method it actually knows about is the addNote
method
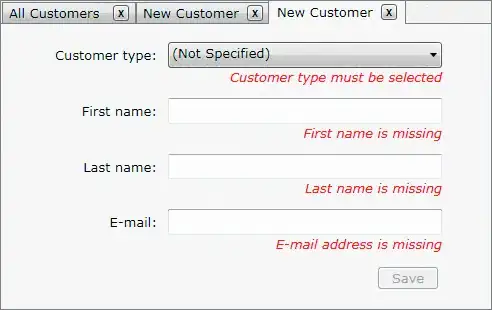
import java.awt.BorderLayout;
import java.awt.Dimension;
import java.awt.EventQueue;
import java.awt.GridBagLayout;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.DateFormat;
import java.util.Date;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextArea;
import javax.swing.UIManager;
import javax.swing.UnsupportedLookAndFeelException;
public class CrossCalling {
public static void main(String[] args) {
new CrossCalling();
}
public CrossCalling() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
NotePadPane notePadPane = new NotePadPane();
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setLayout(new BorderLayout());
frame.add(new SecretaryPane(notePadPane));
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
JFrame noteFrame = new JFrame("Testing");
noteFrame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
noteFrame.setLayout(new BorderLayout());
noteFrame.add(notePadPane);
noteFrame.pack();
noteFrame.setLocation(frame.getX(), frame.getY() + frame.getHeight());
noteFrame.setVisible(true);
}
});
}
public interface NotePad {
public void addNote(String note);
}
public class SecretaryPane extends JPanel {
private NotePad notePad;
public SecretaryPane(NotePad pad) {
this.notePad = pad;
setLayout(new GridBagLayout());
JButton btn = new JButton("Make note");
btn.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
pad.addNote(DateFormat.getTimeInstance().format(new Date()));
}
});
add(btn);
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
}
public class NotePadPane extends JPanel implements NotePad {
private JTextArea ta;
public NotePadPane() {
setLayout(new BorderLayout());
ta = new JTextArea(10, 20);
add(new JScrollPane(ta));
}
@Override
public Dimension getPreferredSize() {
return new Dimension(200, 200);
}
@Override
public void addNote(String note) {
ta.append(note + "\n");
}
}
}