How do I detect that there is a large screen ?
Solution -
Let the application detect if there is a large screen.
- Define different layout files for different screen sizes.
- Ensuring your layout can be adequately resized to fit the screen
- Providing appropriate UI layout according to screen configuration
- Ensuring the correct layout is applied to the correct screen
Read - Multiple Screen Support and Support Screen training docs.
How do I have the main activity load the two fragments into the single activity
if this is the case?
Solution -
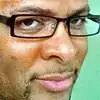
You can combine multiple fragments in a single activity to build a multi-pane UI and reuse a fragment in multiple activities. This is essentially useful when you have defined your fragment container at different layouts. You just need to replace with any other fragment in any layout.
When you navigate to the current layout, you have the id of that container to replace it with the fragment you want.
You can also go back to the previous fragment in the backStack
with the popBackStack()
method. For that you need to add that fragment in the stack using addToBackStack()
and then commit() to reflect. This is in reverse order with the current on top.
Description:
- Let's we want to use two fragments to handle landscape and portrait
modes of the device.
- Next based on number of fragments, create classes which will extend
the Fragment class. The Fragment class has its own callback
functions. You can override any of the functions based on your
requirements.
- Corresponding to each fragment, you will need to create layout files
in XML file. These files will have layout for the defined fragments.
- Finally modify activity file to define the actual logic of replacing
fragments based on your requirement.
Steps to create two fragments in Activity -
1 You will use Eclipse IDE to create an Android application and name it as MyFragments
under a package com.example.myfragments
, with blank Activity.
2 Modify main activity file MainActivity.java
as shown below in the code. Here we will check orientation of the device and accordingly we will switch between different fragments.
3 Create a two java files PM_Fragment.java and LM_Fragement.java under the package com.example.myfragments to define your fragments and associated methods.
4 Create layouts files res/layout/lm_fragment.xml
and res/layout/pm_fragment.xml
and define your layouts for both the fragments.
5 Modify the detault content of res/layout/activity_main.xml
file to include both the fragments.
6 Define required constants in res/values/strings.xml
file
7 Run the application to launch Android emulator and verify the result of the changes done in the aplication.
Read - FragmentTransaction and Android Fragments.