jqGrid don't provide any direct way to customize validation dialog. Nevertheless one can do make some tricks to implement what you need.
First of all one have to examine how jqGrid implements custom validation. jqGrid calls $.jgrid.checkValues
to validate the input data. The method $.jgrid.checkValues
calls custom_func
if required (see here). Then jqGrid calls here $.jgrid.info_dialog
method to display the error message. So one can for example subclass the $.jgrid.info_dialog
method to display custom error message in the way like I described in the answer.
I made the demo which demonstrates the approach. The demo have custom validation in "name" column. The validation require that the saved value have to start with the text "test". I used alert
to display the custom error message. Below is the main parts of the code which I used in the demo:
var useCustomDialog = false, oldInfoDialog = $.jgrid.info_dialog;
...
$.extend($.jgrid,{
info_dialog: function (caption, content, c_b, modalopt) {
if (useCustomDialog) {
// display custom dialog
useCustomDialog = false;
alert("ERROR: " + content);
} else {
return oldInfoDialog.apply (this, arguments);
}
}
});
...
$("#list").jqGrid({
...
colModel: [
{ name: "name", width: 65, editrules: {
custom: true,
custom_func: function (val, nm, valref) {
if (val.slice(0, val.length) === "test") {
return [true];
} else {
useCustomDialog = true; // use custom info_dialog!
return [false, "The name have to start with 'test' text!"];
}
} } },
...
],
...
});
As the result jqGrid could display the error message like below if one would try to save the data for "Clients" ("name") column which text don't start with "test" text
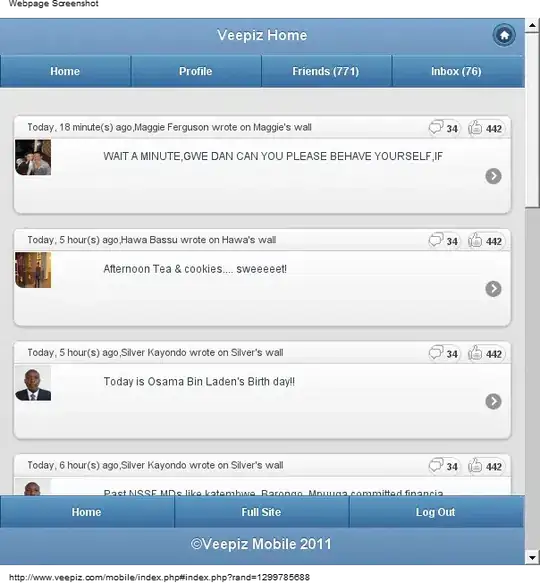