That post that you're referring to I provided an answer to, though it wasn't the accepted answer. I'm surprised I didn't see your previous post you made up until this point, and I sincerely apologize for that.
Normally, when you're doing template matching, you use all of the pixels in your template and you want to use this template to search in your matching image. In that post, the OP had a template, but only wanted to include certain pixels inside the template. The template is a rectangular region that has stuff in it... whether it be grayscale intensities, colour pixels, etc that is a representation of what you're trying to find in the matching image.
In that post, not all of the pixels in this region are to be included in the template. Specifically, only some of the pixels in the template are specified that should be part of the correlation matching. These pixels to be included in the template are specified by a mask, which is what you're looking for in your post. The OP of that post you're referring to wanted to find a circular object ignoring what is inside the actual circle itself.
Therefore, inside the template, they used a mask to extract those pixels that should be part of the correlation matching. Normally, you would do a normalized cross-correlation with all of the pixels in the template with all of the pixels in a region of the same size in the matching image. For that post you're referring to, inside the template, the mask specifies which pixels should be part of the matching. Anything that is white are those pixels that should be included in the matching, while anything that is black should be ignored.
If you look at the mask in the OP's post in this case, it only looks for a circular object itself, disregarding those pixels that are inside the circular object. The contents inside the circular object are not important. They only want to detect the general circular shape. If you were to include these pixels inside the object as part of your template, this may not be able to find the circular object due to some noise that is contained within the object.
The reason why you can't find anything on the topic is because template matching seldom ignores pixels within the template. You want to use as much information as you can in order to try and make your matching more robust. That post you're referring to is a special case that is specific for the OP's application.
Edit
Now that I understand what you are truly after, you want to define a mask programatically. In particular, you want to define the circular mask that was seen in the post you're referring to. This is very simple to code in MATLAB. That circular ring you see has two radii: The inner ring and the outer ring. What you would do is compute the Euclidean distance from the centre of this image to each point in the mask. Those points that are >=
to the radius of the inner ring and <=
to the radius of the outer ring would be set to true
while anything else is set to false
. As such, the general steps are:
- Specify the dimensions of the mask you want, as this will inevitably depend on the size of your template and you want this to match with that size. Also, you need to specify the inner and outer radius of the circular mask.
- Generate a 2D grid of co-ordinates where
(0,0)
is the centre of the the image. Use meshgrid
to do this for you.
- Compute the Euclidean distance from this centre to each point in your image.
- For each distance calculated, check to see if this point is
>=
the inner ring radius and <=
the outer ring radius. If it is, set this to true
, and false
otherwise.
As such:
%// Dimensions of image
%// Change here accordingly
rows = 101;
cols = 101;
%// Radii - Change accordingly
%// Inner ring radius
inner_radius = 20;
%// Outer ring radius
outer_radius = 40;
%// Define grid of points
[X,Y] = meshgrid(-floor(cols/2):floor(cols/2), -floor(rows/2):floor(rows/2));
%// Find Euclidean distance with respect to the centre of the image
dists = sqrt(X.^2 + Y.^2);
%// Find those points that are within the inner and outer radii
out = dists >= inner_radius & dists <= outer_radius;
%// Cap to ensure same mask size
out = out(1:rows,1:cols);
Take special care of the last statement. If you were to specify odd dimensions, then with respect to the centre of the mask, the co-ordinates relative to the centre are correct. For example, if we chose the rows to be 101, then this should span from -50 to 50, including the centre so this exactly corresponds to 101 points. Should we specify...say...100, when using meshgrid
, you will still get co-ordinates from -50 to 50, and so this will still give us a mask of 101 points in the vertical direction (rows). To make sure that you have a template that conforms to the dimensions you specify, we want to be sure to extract out the same amount of rows and columns that you specified in the beginning after you calculate the mask. The difference is only a single pixel border, so it shouldn't really matter... but if you're a stickler and want the same dimensions, then this is what you'll need to do.
If we specify rows = 101, cols = 101, inner_radius = 20, outer_radius = 40
, this is the mask I get:
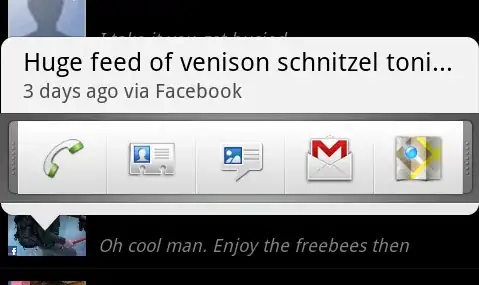