To lay out controls in the requested manner with a GridLayout in SWT you will have to group the controls of each row into a composite of their own like so:
shell.setLayout( new RowLayout( SWT.VERTICAL ) );
Composite composite1 = new Composite( shell, SWT.NONE );
composite1.setLayout( new GridLayout( 2, false ) );
createLabel( composite1, "2020" );
createLabel( composite1, "808080808080" );
Composite composite2 = new Composite( shell, SWT.NONE );
composite2.setLayout( new GridLayout( 2, false ) );
createLabel( composite2, "50505050" );
createLabel( composite2, "50505050" );
private static Label createLabel( Composite parent, String text ) {
Label label = new Label( parent, SWT.NONE );
label.setText( text );
return label;
}
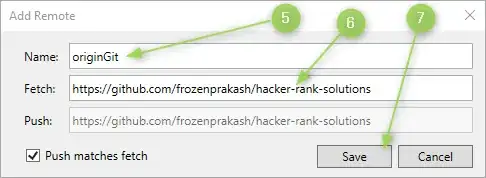
However, to me a FormLayout
seems more suitable to solve the given problem:
shell.setLayout( new FormLayout() );
FormData leftFormData = new FormData();
leftFormData.top = new FormAttachment( 0 );
leftFormData.left = new FormAttachment( 0 );
leftFormData.right = new FormAttachment( 20 );
Label leftLabel = createLabel( shell, "2020", leftFormData );
FormData rightFormData = new FormData();
rightFormData.top = new FormAttachment( 0 );
rightFormData.left = new FormAttachment( leftLabel );
rightFormData.right = new FormAttachment( 100 );
createLabel( shell, "808080808080", rightFormData );
private static Label createLabel( Composite parent, String text, Object layoutData ) {
Label label = new Label( parent, SWT.NONE );
label.setText( text );
label.setLayoutData( layoutData );
return label;
}
If you find the formData and formAttachment code too verbose, you may have a look at Slim Down SWT FormLayout Usage
And for an in-depth discussion of SWT layouts I recommend the Understanding Layouts in SWT article.