Try returning an instance of JMenuBar
from the initializeMenu
and apply it to the Paint
class
public static JMenuBar initializeMenu() {
// JMenu Bar
menuBar = new JMenuBar();
menuBar.setBackground(Color.GRAY);
// JMenu
fileMenu = new JMenu("File");
editMenu = new JMenu("Edit");
menuBar.add(fileMenu);
menuBar.add(editMenu);
// JMenu Items
jItem = new JMenuItem("Save");
fileMenu.add(jItem);
return menuBar;
}
Then apply it yo the Paint
class...
public static void main(String[] args) {
SwingUtilities.invokeLater(new Runnable() {
public void run() {
Paint paint = new Paint();
paint.setJMenuBar(initializeMenu());
paint.setLayout(new FlowLayout());
paint.setSize(800, 500);
paint.setVisible(true);
}
});
}
Personally, I'd favour pack
over setSize
, it will generally result in a viewable area which will meet your needs (assuming you're using the layout management API properly)
Updated...
You've broken the paint chain
public class Paint extends JFrame implements ... {
//...
// Method for different drawing stencils
public void paint(Graphics g) {
if (p != null) {
g.setColor(c);
switch (shapeType) {
case 0:
g.drawOval(p.x - w / 2, p.y - h / 2, w, h);
break;
case 1:
g.drawRect(p.x - w / 2, p.y - h / 2, w, h);
break;
}
}
// Resets application window surface to white (clears the canvas)
if (Refresh == true) {
g.setColor(Color.white);
g.fillRect(0, 0, 1500, 1500);
}
g.drawImage(key, 0, 0, this);
if (widthincrease == true) {
w += 1;
}
if (heightincrease == true) {
h += 1;
}
if (widthdecrease == true) {
w -= 1;
if (w < 1) {
w = 50;
}
}
if (heightdecrease == true) {
h -= 1;
if (h < 1) {
h = 50;
}
}
try {
Thread.sleep(10);
} catch (InterruptedException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
repaint();
}
public void update(Graphics g) {
paint(g);
}
}
Basically, by overriding paint
, but never calling super.paint
, you've prevented the frame from ever painting it's content.
First, you should avoid overriding paint
of top level containers, this is just one example of way, but JFrame
has a bunch of other components which reside on top it.
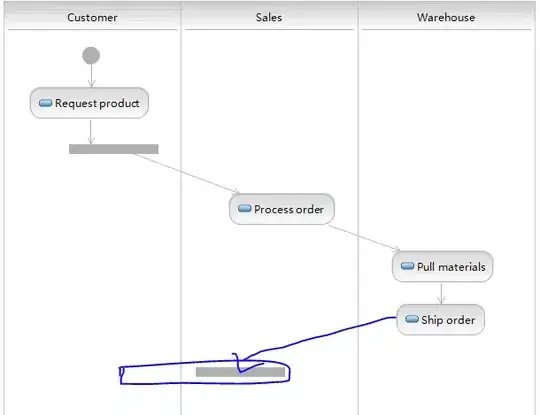
These can (and will) paint over anything you are trying to paint to the frame.
The frame has borders, which are paint within the frames area, by overriding paint
, you can paint under these borders, see How to get the EXACT middle of a screen, even when re-sized for an example of what I'm talking about.
Instead, create a custom class that extends from JPanel
and override it's paintComponent
method, move the rest of the "painting" related to code this class (and don't forget to call super.paintComponent
before doing any custom painting).
See Performing Custom Painting for more details
Avoid KeyListener
, seriously, it's just a paint, instead, use the key bindings API, see How to Use Key Bindings for more details