The regional formatting behaves correctly (IMHO) in the Windows Phone Silverlight stack but misbehaves (still IMHO) in the WinRT stack. It has been misbehaving since the Windows 8.0 and there's lengthy discussion about the issue here: WinRT apps and Regional settings. The correct way to format dates and numbers based on the user's regional settings?
Also I did a little more recent blog post about the issue here: http://mikaelkoskinen.net/winrt-xaml-misbehave-still-in-windows-81/
The blog post also contains a workaround which seems to work OK: You can set the Windows.Globalization.ApplicationLanguages.PrimaryLanguageOverride to the user's regional format to make things work better.
So, hopefully the following code in your App.xaml.cs's constructor proves useful:
public App()
{
Windows.Globalization.ApplicationLanguages.PrimaryLanguageOverride = Windows.System.UserProfile.GlobalizationPreferences.HomeGeographicRegion;
this.InitializeComponent();
this.Suspending += this.OnSuspending;
}
Updated with alternative version
Another alternative is to use GlobalizationPreferences.Languages-array. For example:
Windows.Globalization.ApplicationLanguages.PrimaryLanguageOverride = Windows.System.UserProfile.GlobalizationPreferences.Languages[1];
Just make sure to check that there actually is more than 1 language in the array.
This is how it looks in the app after setting the PrimaryLanguageOverride:
this.DateText.Text = DateTime.Now.ToString("D");
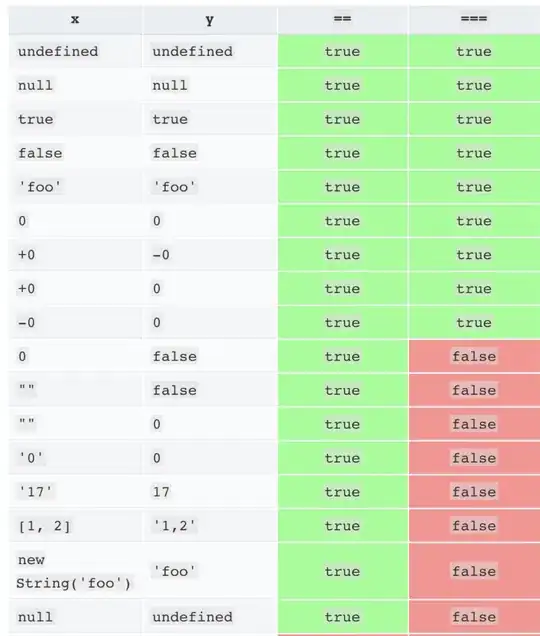
Updated with screenshots
In Windows Phone 8.1, if you change the Regional format through Settings, the selected Regional format will be added as the the second language. At least this how the 8.1 emulator works. So, when I start the emulator from scratch and change the regional setting:
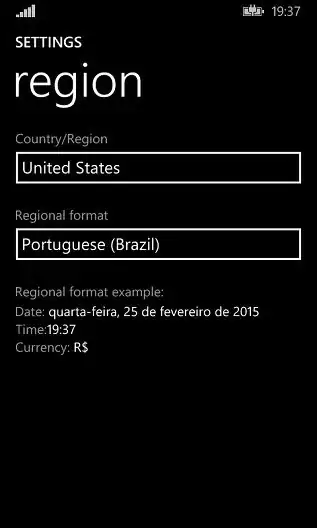
And then if I restart the phone, the selected region has been added as the second language:
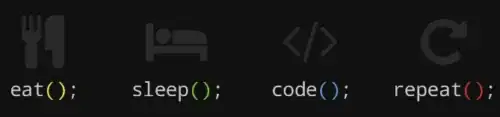
And now the Windows.System.UserProfile.GlobalizationPreferences.Languages contains the Region format I selected.