There are a lot of details that would go into answering this question.
The first would be, what have you got so far?
Do you have a map image?
Have you plotted the gps coordinates to coincide with the pixel positions in your image?
Do you have a Look Up Table to correspond with your different "temp" values?
Are you looking to create a static output image, or do you want it to update dynamically? (This may determine the method you use to generate the heat-map overlay.)
Once you have those details ironed out it should be fairly simple to generate a heat map like the one above using any of the various imaging libraries available in Python (PIL/OpenCV).
This is a rough (overly simplified) outline of how I would generate the heat map from the initial data using OpenCV:
I would start with two images, the map image, and a zero valued (black) image of the same size. You can then add the appropriate values to all three color channels of your blank image at the gps/pixel locations which will give you a 3 channeled gray image. (So if the value is 0.25, you set RG and B to 0.25)

Then, apply a gaussian blur with a large kernel size; appropriate to the amount of blending you want between points and the size of your image.
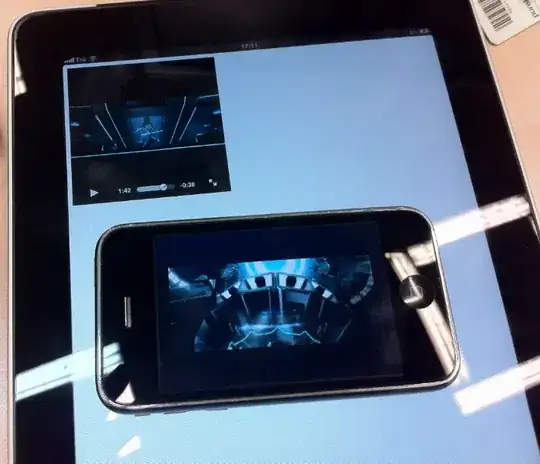
You will likely need to multiply your blurred image by some factor (depending on kernel size) after blurring to brighten the colors.

Next apply your Look Up Table to your Blurred mapped values:
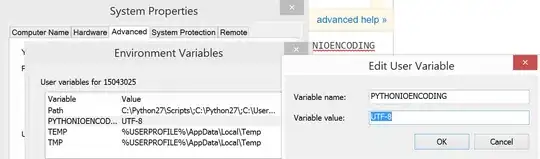
Then you could merge the two images into one output image using any number of combinations (add, multiply, addWeighted(), etc). Or if you want to involve an alpha channel for a cleaner overlay you could use the method described here.
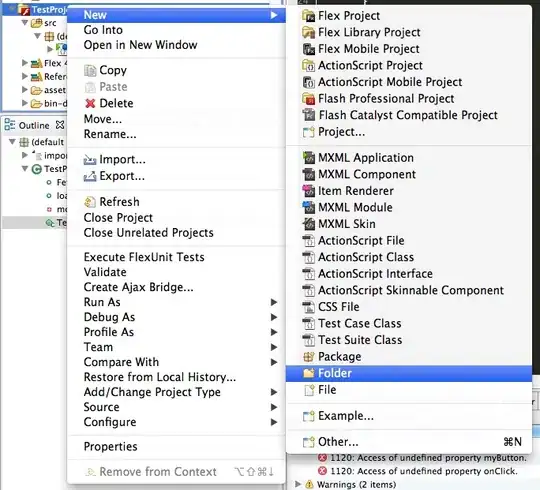