Here is a full example using rich text of QTextDocument.
mainWindow.cpp:
#include "mainWindow.h"
void MainWindow::paintEvent(QPaintEvent*)
{
QPainter painter(this);
QTextDocument td;
td.setHtml("K<sub>max</sub>=K<sub>2</sub> · 3");
td.drawContents(&painter);
}
If you need to draw the text at specific point, translate the coordinate system of the painter before drawing:
painter.translate(QPointF(50, 50));
mainWindow.cpp - Another solution:
#include "mainWindow.h"
void MainWindow::paintEvent(QPaintEvent*)
{
QPainter painter(this);
QTextDocument td;
td.setHtml("K<sub>max</sub>=K<sub>2</sub> · 3");
QAbstractTextDocumentLayout::PaintContext ctx;
ctx.clip = QRectF( 0, 0, 400, 100 );
td.documentLayout()->draw( &painter, ctx );
}
mainWindow.h:
#include <QtGui>
class MainWindow: public QWidget
{
protected:
void paintEvent(QPaintEvent*);
};
main.cpp:
#include <QtGui>
#include "mainWindow.h"
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
MainWindow mainWindow;
mainWindow.show();
return app.exec();
}
The project file:
TEMPLATE = app
QT += gui
HEADERS = mainWindow.h
SOURCES = main.cpp mainWindow.cpp
Result:
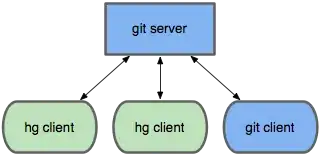
Kmax
=K2
.3"); lbl.show();`. – vahancho Apr 27 '15 at 09:59