as @berak pointed out, you can use moments to find centroid of blobs
#include <opencv2/highgui/highgui.hpp>
#include <opencv2/imgproc/imgproc.hpp>
using namespace cv;
Point getCentroid( InputArray Points )
{
Point Coord;
Moments mm = moments( Points, false );
double moment10 = mm.m10;
double moment01 = mm.m01;
double moment00 = mm.m00;
Coord.x = int(moment10 / moment00);
Coord.y = int(moment01 / moment00);
return Coord;
}
int main( int argc, char* argv[] )
{
Mat src = imread( argv[1] );
Mat gray;
cvtColor( src, gray, CV_BGR2GRAY );
gray = gray > 127;
Mat nonzeropixels;
findNonZero( gray, nonzeropixels );
Point pt = getCentroid( nonzeropixels );
rectangle(src, pt,pt, Scalar(0, 255, 0), 2);
imshow("result",src);
waitKey();
return 0;
}
test images & results : ( green dot is centroid of white blobs )

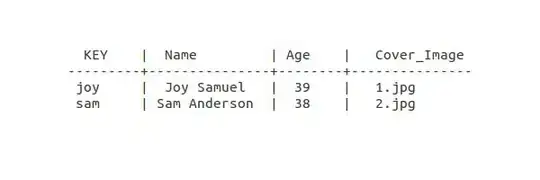