You can't detect that case, because application is not open using push notification (it has been open via app icon).
Try to open application by swiping push notification.
EDIT:
If you want to be invoked for push notification (via background fetch, when your application is not active) you need to ask your backend developer to set "content-available": 1
in push notification.
After that -application:didReceiveRemoteNotification:fetchCompletionHandler:
will be invoked (when push-notification arrives), so you can save the payload into a file and then when application will be open, you can read the file and take actions.
- (void)application:(UIApplication *)application
didReceiveRemoteNotification:(NSDictionary *)userInfo
fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler
{
NSLog(@"#BACKGROUND FETCH CALLED: %@", userInfo);
// When we get a push, just writing it to file
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString *filePath = [documentsDirectory stringByAppendingPathComponent:@"userInfo.plist"];
[userInfo writeToFile:filePath atomically:YES];
completionHandler(UIBackgroundFetchResultNewData);
}
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
// Checking if application was launched by tapping icon, or push notification
if (!launchOptions[UIApplicationLaunchOptionsRemoteNotificationKey]) {
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
NSString *documentsDirectory = [paths objectAtIndex:0];
NSString *filePath = [documentsDirectory stringByAppendingPathComponent:@"userInfo.plist"];
[[NSFileManager defaultManager] removeItemAtPath:filePath
error:nil];
NSDictionary *info = [NSDictionary dictionaryWithContentsOfFile:filePath];
if (info) {
// Launched by tapping icon
// ... your handling here
}
} else {
NSDictionary *info = launchOptions[UIApplicationLaunchOptionsRemoteNotificationKey];
// Launched with swiping
// ... your handling here
}
return YES;
}
Also don't forget to enable "Remote notifications" in "Background Modes"
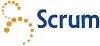