I made an example of "Angular - Use images like checkboxes and radios"
Link to Stackblitz
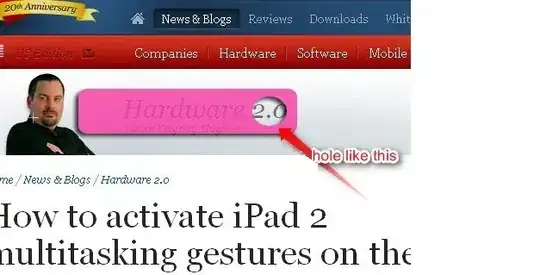
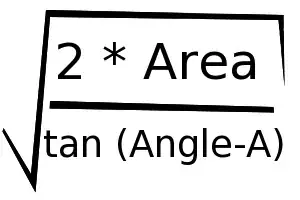
In app.component.html file:
<div class="cont-bg">
<h5 class="text-white">Checkbox</h5>
<div class="cont-main">
<div class="cont-checkbox" *ngFor="let car of cars; index as i">
<input type="checkbox" [id]="'myCheckbox-' + i" />
<label [for]="'myCheckbox-' + i">
<img [src]="car.img" />
<span class="cover-checkbox">
<svg viewBox="0 0 12 10">
<polyline points="1.5 6 4.5 9 10.5 1"></polyline>
</svg>
</span>
<div class="info">{{ car.name }}</div>
</label>
</div>
</div>
<h5 class="text-white">Radio</h5>
<div class="cont-main">
<div class="cont-checkbox" *ngFor="let car of cars; index as i">
<input type="radio" name="myRadio" [id]="'myRadio-' + i" />
<label [for]="'myRadio-' + i">
<img [src]="car.img" />
<span class="cover-checkbox">
<svg viewBox="0 0 12 10">
<polyline points="1.5 6 4.5 9 10.5 1"></polyline>
</svg>
</span>
<div class="info">{{ car.name }}</div>
</label>
</div>
</div>
</div>
In app.component.scss file:
* {
font-family: Lato;
--transition: 0.15s;
--border-radius: 0.5rem;
--background: #ffc107;
--box-shadow: #ffc107;
// --box-shadow: #0082ff;
}
.cont-bg {
min-height: 100vh;
background-image: radial-gradient(
circle farthest-corner at 7.2% 13.6%,
rgba(37, 249, 245, 1) 0%,
rgba(8, 70, 218, 1) 90%
);
padding: 1rem;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.cont-main {
display: flex;
flex-wrap: wrap;
align-content: center;
justify-content: center;
}
.cont-checkbox {
width: 150px;
height: 100px;
border-radius: var(--border-radius);
box-shadow: 0 0.125rem 0.25rem rgba(0, 0, 0, 0.075);
transition: transform var(--transition);
background: white;
margin-bottom: 0.75rem;
margin-right: 0.75rem;
&:active {
transform: scale(0.9);
}
input {
display: none;
&:checked + label {
opacity: 1;
box-shadow: 0 0 0 3px var(--background);
.cover-checkbox {
opacity: 1;
transform: scale(1);
svg {
stroke-dashoffset: 0;
}
}
img {
-webkit-filter: none; /* Safari 6.0 - 9.0 */
filter: none;
}
}
}
label {
cursor: pointer;
border-radius: var(--border-radius);
overflow: hidden;
width: 100%;
height: 100%;
position: relative;
opacity: 0.6;
img {
width: 100%;
height: 70%;
object-fit: cover;
clip-path: polygon(0% 0%, 100% 0, 100% 81%, 50% 100%, 0 81%);
-webkit-filter: grayscale(100%); /* Safari 6.0 - 9.0 */
filter: grayscale(100%);
}
.cover-checkbox {
position: absolute;
right: 5px;
top: 3px;
z-index: 1;
width: 23px;
height: 23px;
border-radius: 50%;
background: var(--box-shadow);
border: 2px solid #fff;
transition: transform var(--transition),
opacity calc(var(--transition) * 1.2) linear;
opacity: 0;
transform: scale(0);
svg {
width: 13px;
height: 11px;
display: inline-block;
vertical-align: top;
fill: none;
margin: 5px 0 0 3px;
stroke: #fff;
stroke-width: 2;
stroke-linecap: round;
stroke-linejoin: round;
stroke-dasharray: 16px;
transition: stroke-dashoffset 0.4s ease var(--transition);
stroke-dashoffset: 16px;
}
}
.info {
text-align: center;
margin-top: 0.2rem;
font-weight: 600;
font-size: 0.8rem;
}
}
}
In app.component.ts file:
import { Component, VERSION } from '@angular/core';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: ['./app.component.scss'],
})
export class AppComponent {
cars = [
{
id: '1',
name: 'Mazda MX-5 Miata',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-mazda-mx-5-miata-mmp-1-1593459650.jpg?crop=0.781xw:0.739xh;0.109xw,0.0968xh&resize=480:*',
},
{
id: '2',
name: 'Toyota Supra',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2020-chevrolet-corvette-c8-102-1571146873.jpg?crop=0.548xw:0.411xh;0.255xw,0.321xh&resize=980:*',
},
{
id: '3',
name: 'Chevy Corvette',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-porsche-cayman-mmp-1-1593003674.jpg?crop=0.648xw:0.485xh;0.129xw,0.263xh&resize=980:*',
},
{
id: '4',
name: 'Porsche 718 Cayman',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-porsche-718-cayman-mmp-1-1593003156.jpg?crop=0.735xw:0.551xh;0.138xw,0.240xh&resize=980:*',
},
{
id: '5',
name: 'Porsche 718 Boxster',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-bmw-m2-mmp-1-1599687968.jpg?crop=0.706xw:0.528xh;0.102xw,0.268xh&resize=980:*',
},
{
id: '6',
name: 'BMW M2',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-bmw-z4-mmp-1-1599583762.jpg?crop=0.779xw:0.584xh;0.0782xw,0.196xh&resize=980:*',
},
{
id: '7',
name: 'BMW Z4',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-chevrolet-camaro-mmp-1-1585333717.jpg?crop=0.344xw:0.331xh;0.241xw,0.328xh&resize=980:*',
},
{
id: '8',
name: 'Chevy Camaro',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-chevrolet-camaro-zl1-mmp-1-1604071262.jpg?crop=0.818xw:0.663xh;0.0799xw,0.163xh&resize=980:*',
},
{
id: '9',
name: 'Chevy Camaro ZL1',
img: 'https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-chevrolet-camaro-zl1-mmp-1-1604071262.jpg?crop=0.818xw:0.663xh;0.0799xw,0.163xh&resize=768:*',
},
];
}
Pure CSS Checkbox and Radio:
* {
font-family: Lato;
margin: 0;
padding: 0;
--transition: 0.15s;
--border-radius: 0.5rem;
--background: #ffc107;
--box-shadow: #ffc107;
}
.cont-bg {
min-height: 100vh;
background-image: radial-gradient(
circle farthest-corner at 7.2% 13.6%,
rgba(37, 249, 245, 1) 0%,
rgba(8, 70, 218, 1) 90%
);
padding: 1rem;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.cont-title {
color: white;
font-size: 1.25rem;
font-weight: 600;
margin-bottom: 1rem;
}
.cont-main {
display: flex;
flex-wrap: wrap;
align-content: center;
justify-content: center;
}
.cont-checkbox {
width: 150px;
height: 100px;
border-radius: var(--border-radius);
box-shadow: 0 0.125rem 0.25rem rgba(0, 0, 0, 0.075);
background: white;
transition: transform var(--transition);
}
.cont-checkbox:first-of-type {
margin-bottom: 0.75rem;
margin-right: 0.75rem;
}
.cont-checkbox:active {
transform: scale(0.9);
}
input {
display: none;
}
input:checked + label {
opacity: 1;
box-shadow: 0 0 0 3px var(--background);
}
input:checked + label img {
-webkit-filter: none; /* Safari 6.0 - 9.0 */
filter: none;
}
input:checked + label .cover-checkbox {
opacity: 1;
transform: scale(1);
}
input:checked + label .cover-checkbox svg {
stroke-dashoffset: 0;
}
label {
display: inline-block;
cursor: pointer;
border-radius: var(--border-radius);
overflow: hidden;
width: 100%;
height: 100%;
position: relative;
opacity: 0.6;
}
label img {
width: 100%;
height: 70%;
object-fit: cover;
clip-path: polygon(0% 0%, 100% 0, 100% 81%, 50% 100%, 0 81%);
-webkit-filter: grayscale(100%); /* Safari 6.0 - 9.0 */
filter: grayscale(100%);
}
label .cover-checkbox {
position: absolute;
right: 5px;
top: 3px;
z-index: 1;
width: 20px;
height: 20px;
border-radius: 50%;
background: var(--box-shadow);
border: 2px solid #fff;
transition: transform var(--transition),
opacity calc(var(--transition) * 1.2) linear;
opacity: 0;
transform: scale(0);
}
label .cover-checkbox svg {
width: 13px;
height: 11px;
display: inline-block;
vertical-align: top;
fill: none;
margin: 5px 0 0 3px;
stroke: #fff;
stroke-width: 2;
stroke-linecap: round;
stroke-linejoin: round;
stroke-dasharray: 16px;
transition: stroke-dashoffset 0.4s ease var(--transition);
stroke-dashoffset: 16px;
}
label .info {
text-align: center;
margin-top: 0.2rem;
font-weight: 600;
font-size: 0.8rem;
}
<div class="cont-bg">
<div class="cont-title">Checkbox</div>
<div class="cont-main">
<div class="cont-checkbox">
<input type="checkbox" id="myCheckbox-1" />
<label for="myCheckbox-1">
<img
src="https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-mazda-mx-5-miata-mmp-1-1593459650.jpg?crop=0.781xw:0.739xh;0.109xw,0.0968xh&resize=480:*"
/>
<span class="cover-checkbox">
<svg viewBox="0 0 12 10">
<polyline points="1.5 6 4.5 9 10.5 1"></polyline>
</svg>
</span>
<div class="info">Mazda MX-5 Miata</div>
</label>
</div>
<div class="cont-checkbox">
<input type="checkbox" id="myCheckbox-2" />
<label for="myCheckbox-2">
<img
src="https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2020-chevrolet-corvette-c8-102-1571146873.jpg?crop=0.548xw:0.411xh;0.255xw,0.321xh&resize=980:*"
/>
<span class="cover-checkbox">
<svg viewBox="0 0 12 10">
<polyline points="1.5 6 4.5 9 10.5 1"></polyline>
</svg>
</span>
<div class="info">Toyota Supra</div>
</label>
</div>
</div>
<div class="cont-title">Radio</div>
<div class="cont-main">
<div class="cont-checkbox">
<input type="radio" name="myRadio" id="myRadio-1" />
<label for="myRadio-1">
<img
src="https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2021-mazda-mx-5-miata-mmp-1-1593459650.jpg?crop=0.781xw:0.739xh;0.109xw,0.0968xh&resize=480:*"
/>
<span class="cover-checkbox">
<svg viewBox="0 0 12 10">
<polyline points="1.5 6 4.5 9 10.5 1"></polyline>
</svg>
</span>
<div class="info">Mazda MX-5 Miata</div>
</label>
</div>
<div class="cont-checkbox">
<input type="radio" name="myRadio" id="myRadio-2" />
<label for="myRadio-2">
<img
src="https://hips.hearstapps.com/hmg-prod.s3.amazonaws.com/images/2020-chevrolet-corvette-c8-102-1571146873.jpg?crop=0.548xw:0.411xh;0.255xw,0.321xh&resize=980:*"
/>
<span class="cover-checkbox">
<svg viewBox="0 0 12 10">
<polyline points="1.5 6 4.5 9 10.5 1"></polyline>
</svg>
</span>
<div class="info">Toyota Supra</div>
</label>
</div>
</div>
</div>