Sometimes I go crazy and pick up a challenge just for fun :-)
Here is the result:
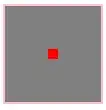
I overlay the TabControl tabControl1
with a Panel tp
. (Do pick better names!) Note that this must happen rather late or else the TabControl
will pop to the top.
I call them to order in the Shown
event:
private void Form1_Shown(object sender, EventArgs e)
{
tp.BringToFront();
tabControl1.SendToBack();
}
You probably could create the Panel
in the designer to avoid these motions..
In addition to the Panel
I need a List<GraphicsPath>
which is used both for drawing and for precise hit testing..:
Panel tp = null;
List<GraphicsPath> tabAreas = new List<GraphicsPath>();
You can call this function to prepare both the Panel
and the List
:
void makeTabPanel(TabControl tab)
{
tp = new Panel();
tp.Size = new Size(tab.Width, tab.ItemSize.Height);
tp.Paint += tp_Paint;
tp.MouseClick += tp_MouseClick;
tp.Location = tab.Location;
tab.Parent.Controls.Add(tp);
int tabs = tabControl1.TabPages.Count;
float w = tabControl1.Width / tabs;
float h = tp.Size.Height;
float y0 = 0; float y1 = h / 2f; float y2 = h;
float d = 5; // <--- this is the gap
float e = 8; // <- this is the extrusion
float w1 = w - d;
tabAreas = new List<GraphicsPath>();
for (int t = 0; t < tabs; t++)
{
int t1 = t + 1;
float e1 = t == 0 ? 0 : e; // corrections for start and end..
float e2 = t == tabs - 1 ? 0 : e;
float e3 = t == tabs - 1 ? d : 0;
List<PointF> points = new List<PointF>();
points.Add(new PointF(t * w, y0));
points.Add(new PointF(t1 * w - d + e3, y0));
points.Add(new PointF(t1 * w -d + e2 + e3, y1));
points.Add(new PointF(t1 * w- d + e3, y2));
points.Add(new PointF(t * w, y2));
points.Add(new PointF(t * w + e1, y1));
GraphicsPath gp = new GraphicsPath(FillMode.Alternate);
gp.AddPolygon(points.ToArray());
tabAreas.Add(gp);
}
}
After this the actual events are rather simple:
void tp_MouseClick(object sender, MouseEventArgs e)
{
for (int t = 0; t < tabAreas.Count; t++)
{
if (tabAreas[t].IsVisible(e.Location) )
{ tabControl1.SelectedIndex = t; break;}
}
tp.Invalidate();
}
void tp_Paint(object sender, PaintEventArgs e)
{
StringFormat fmt = new StringFormat()
{ Alignment = StringAlignment.Center, LineAlignment = StringAlignment.Center };
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias;
e.Graphics.Clear(Color.White); // **
float w = tp.Width / tabAreas.Count;
Size sz = new System.Drawing.Size( (int)w, e.ClipRectangle.Height);
for (int t = 0; t < tabAreas.Count; t++)
{
Rectangle rect = new Rectangle((int)(t * w ), 0, sz.Width, sz.Height);
bool selected = tabControl1.SelectedIndex == t ;
Brush brush = selected ? Brushes.DarkGoldenrod : Brushes.DarkGray; // **
e.Graphics.FillPath(brush, tabAreas[t]);
e.Graphics.DrawString(tabControl1.TabPages[t].Text,
tabControl1.Font, Brushes.White, rect, fmt);
}
}
Pick your colors here (**)
Update: Using TextRenderer as Lars suggests works just as well. (At least ;-)
TextFormatFlags flags = TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter ;
TextRenderer.DrawText(e.Graphics, tabControl1.TabPages[t].Text,
tabControl1.Font, rect, Color.White, flags);
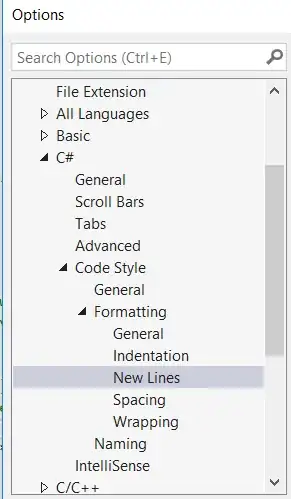