TLDR — Here is some usage guidance, including some methods that haven't been mentioned yet:
Use case |
Recommended |
Example |
Speed |
DataFrame.loc |
df.loc[df['A'] < 10, 'A'] = 1 |
Method chaining |
Series.mask |
df['A'] = df['A'].mask(df['A'] < 10, 1).method1().method2() |
Whole dataframe |
DataFrame.mask |
df = df.mask(df['A'] < 10, df**2) |
Multiple conditions |
np.select |
df['A'] = np.select([df['A'] < 10, df['A'] > 20], [1, 2], default=df['A']) |
1. Speed
Use DataFrame.loc
if you have a large dataframe and are concerned about speed:
df.loc[df['Season'] > 1990, 'Season'] = 1
For small dataframes, speed is trivial, but technically there are faster options if you want:
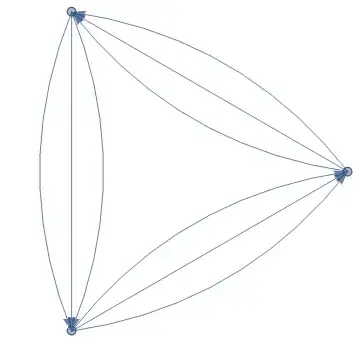
df = pd.DataFrame({'Team': np.random.choice([*'ABCDEFG'], size=n), 'Season': np.random.randint(1900, 2001, size=n), 'Games': np.random.randint(0, 17, size=n)})
2. Method chaining
Use a Series
method if you want to conditionally replace values within a method chain:
Series.mask
replaces values where the given condition is true
df['Season'] = df['Season'].mask(df['Season'] > 1990, 1)
Series.where
is just the inverted version (replace when false)
df['Season'] = df['Season'].where(df['Season'] <= 1990, 1)
The chaining benefit is not obvious in OP's example but is very useful in other situations. Just as a toy example:
# compute average games per team, but pre-1972 games are weighted by half
df['Games'].mask(df['Season'] < 1972, 0.5*df['Games']).groupby(df['Team']).mean()
Practical examples:
3. Whole dataframe
Use DataFrame.mask
if you want to conditionally replace values throughout the whole dataframe.
It's not easy to come up with a meaningful example given OP's sample, but here is a trivial example for demonstration:
# replace the given elements with the doubled value (or repeated string)
df.mask(df.isin(['Chicago Bears', 'Buffalo Bills', 8, 1990]), 2*df)
Practical example:
4. Multiple conditions
Use np.select
if you have multiple conditions, each with a different replacement:
# replace pre-1920 seasons with 0 and post-1990 seasons with 1
conditions = {
0: df['Season'] < 1920,
1: df['Season'] > 1990,
}
df['Season'] = np.select(conditions.values(), conditions.keys(), default=df['Season'])
Practical example: