Personally, I'd avoid using a JLabel
for this purpose, it does not calculate it's required size based on it's content, but rather off it's icon
and text
properties.
This might be a good or bad thing, but it can catch your unawares if you're not aware of it.
Instead, I'd use a customised JPanel
, which would allow you to define things like the resize and fill rules, for example and for example
Now, once you have that covered, you need to create a panel of your buttons. I prefer to create a dedicated class, as it makes it easier to isolate functionality and management, but that's me...
public class ButtonPane extends JPanel {
public ButtonPane() {
setLayout(new GridBagLayout());
setBorder(new EmptyBorder(8, 8, 8, 8));
GridBagConstraints gbc = new GridBagConstraints();
gbc.gridwidth = GridBagConstraints.REMAINDER;
gbc.insets = new Insets(2, 2, 2, 2);
add(new JButton("Button 1"), gbc);
add(new JButton("Button 2"), gbc);
add(new JButton("Button 3"), gbc);
}
}
Next, you need to add this panel to your background
JFrame frame = new JFrame("Testing");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setContentPane(backgroundPane);
frame.setLayout(new GridBagLayout());
GridBagConstraints gbc = new GridBagConstraints();
gbc.weightx = 1;
gbc.weighty = 1;
gbc.anchor = GridBagConstraints.SOUTHEAST;
gbc.insets = new Insets(30, 30, 30, 30);
ButtonPane buttonPane = new ButtonPane();
frame.add(buttonPane, gbc);
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
Which can generate something like...
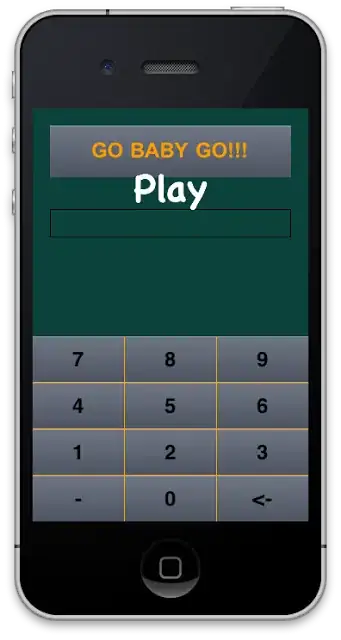
Have a look at Laying Out Components Within a Container and How to Use GridBagLayout for some more details