You have a resource location problem.
Toolkit#createImage
may return an empty image if the resource can not be found.
I suggest you use the ImageIO
API instead, it supports a wider range of image formats, but will also throw an exception if the image is not found or can not be loaded.
How you load the image will also depend on where the image is.
If the image exists on the file system, you can simply use a File
object reference, if the image is an embedded resource (within you application), you will need to use Class#getResource
to obtain a URL
to it.
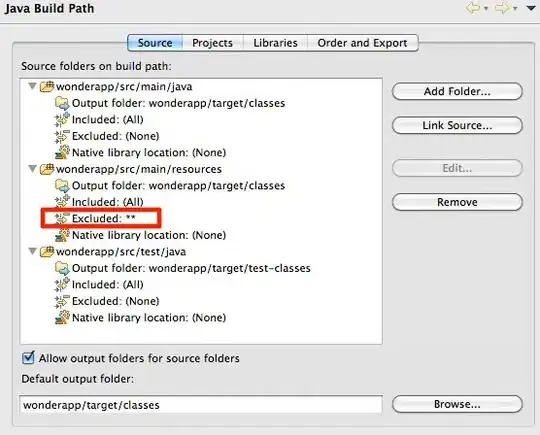
public class TestGraphics {
public static void main(String[] args) {
new TestGraphics();
}
public TestGraphics() {
EventQueue.invokeLater(new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (ClassNotFoundException | InstantiationException | IllegalAccessException | UnsupportedLookAndFeelException ex) {
}
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setContentPane(new PaintTest());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
});
}
public class PaintTest extends JPanel {
private BufferedImage image;
public PaintTest() {
setLayout(new BorderLayout());
try {
// Use this if the image exists within the file system
image = ImageIO.read(new File("/path/to/image/imageName.png"));
// Use this if the image is an embedded resource
// image = ImageIO.read(getClass().getResource("/path/to/resource/imageName.png"));
} catch (Exception e) {
e.printStackTrace();
}
}
@Override
public Dimension getPreferredSize() {
return image == null ? super.getPreferredSize() : new Dimension (image.getWidth(), image.getHeight());
}
@Override
protected void paintComponent(Graphics g) {
super.paintComponent(g);
if (image != null) {
int x = (getWidth() - image.getWidth()) / 2;
int y = (getHeight()- image.getHeight()) / 2;
g.drawImage(image, x, y, this);
}
}
}
}