Based on ChartJS: Change the positions of the tooltips
Preview
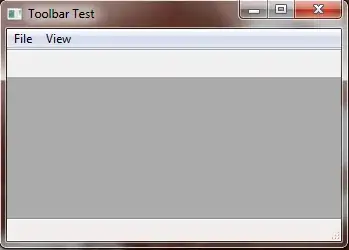
Converting the above to angular-chart is easy because we are only setting the options. However we need to make 2 minor changes (i.e. set chart and ctx variables from this
// THIS IS REQUIRED AND WAS ADDED
tooltipEvents: [],
onAnimationComplete: function () {
// THESE 2 LINES ARE NEW
var chart = this.chart;
var ctx = this.chart.ctx;
this.segments.forEach(function (segment) {
var outerEdge = Chart.Arc.prototype.tooltipPosition.apply({
// THESE 2 LINES WERE CHANGED
x: chart.width / 2,
y: chart.height / 2,
startAngle: segment.startAngle,
endAngle: segment.endAngle,
outerRadius: segment.outerRadius * 2 + 10,
innerRadius: 0
})
...
The entire code assuming you have the library scripts included looks something like this
HTML
<div ng-app="myApp">
<div ng-controller="myController">
<canvas id="polar-area" class="chart chart-polar-area" data="data" labels="labels" options="options"></canvas>
</div>
</div>
Script
angular.module('myApp', ["chart.js"]);
angular.module('myApp').controller('myController', function ($scope) {
$scope.labels = ["Download Sales", "In-Store Sales", "Mail-Order Sales", "Tele Sales", "Corporate Sales"];
$scope.data = [300, 500, 100, 40, 120];
$scope.options = {
scaleOverride: true,
scaleStartValue: 0,
scaleStepWidth: 40,
scaleSteps: 10,
tooltipEvents: [],
onAnimationComplete: function () {
var chart = this.chart;
var ctx = this.chart.ctx;
this.segments.forEach(function (segment) {
var outerEdge = Chart.Arc.prototype.tooltipPosition.apply({
x: chart.width / 2,
y: chart.height / 2,
startAngle: segment.startAngle,
endAngle: segment.endAngle,
outerRadius: segment.outerRadius * 2 + 10,
innerRadius: 0
})
var normalizedAngle = (segment.startAngle + segment.endAngle) / 2;
while (normalizedAngle > 2 * Math.PI) {
normalizedAngle -= (2 * Math.PI)
}
if (normalizedAngle < (Math.PI * 0.4) || (normalizedAngle > Math.PI * 1.5))
ctx.textAlign = "start";
else if (normalizedAngle > (Math.PI * 0.4) && (normalizedAngle < Math.PI * 0.6)) {
outerEdge.y += 5;
ctx.textAlign = "center";
}
else if (normalizedAngle > (Math.PI * 1.4) && (normalizedAngle < Math.PI * 1.6)) {
outerEdge.y - 5;
ctx.textAlign = "center";
}
else
ctx.textAlign = "end";
ctx.fillText(segment.label, outerEdge.x, outerEdge.y);
})
}
}
}
);
Fiddle - http://jsfiddle.net/tmzpy7Lt/