You can think of a constructor as like a blueprint.
In the above picture, we have the class in the dotted box. This is the "blueprint." It's not an actual object yet, it just represents how objects should look. In the solid box is an actual object.
The constructor takes the blueprint and actually builds something from it.
Household(String choice,boolean isOn) {
this.isOn=isOn;
this.choice=choice;
}
Here, we're defining how that blueprint works. In order to build a household object, we need to supply it with a string "choice" and a boolean "isOn".
Once we have those two things, we can create a Household object.
Now, you can't turn on the lights in your house before they're built.
Once you have a Household object, you'll also have your choice and isOn fields.
Say I want to turn off whatever is in the house.
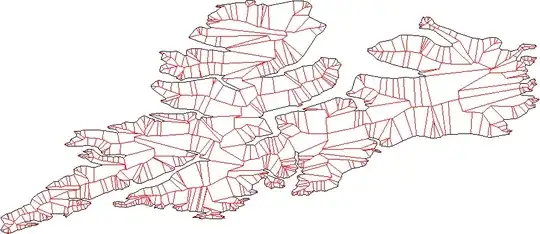
Using your code, we have a household named hh, a toaster, and an on/off switch. If you wanted to turn off the on/off switch, you'd use the setter:
public void setOn(boolean isOn) {
this.isOn = isOn;
}
The setter then sets the value of the isOn field on the hh object, as seen in the above picture.
If you wanted to know whether that switch was true or false (on or off), you'd ask:
hh.getOn();
This returns a boolean value, the same value as the isOn field on the object.
You can see this when you call
System.out.println(hh.getOn());
This prints out true or false, depending on whether or not it's set to true or false.
That's how setters and getters work: the setter sets the value of that field, and the getter gets the value of the field.
Now for the (more) fun part.
Since you want to input data, rather than simply outputting data, we have to do something different.
Say I want to have a user type into the console "off" and have it set the households on/off switch to off.
System.out.println(hh); <-- only prints out to console
We need a new object to handle input. Scanner is commonly used for teaching beginners, so we'll use that.
Scanner s = new Scanner(System.in);
This reads data from the system's input stream. You can read about Scanner here.
Now, scanner reads data in as a string using nextLine();
So we'll use that.
String test = s.nextLine();
And of course now we need to test if that string is the same as "off", and if it is, set the isOn field to false (off).
if(test.equals("off") || test.equals("OFF")){
hh.setOn(false);
}
I won't go into more details on how to do the rest of this, such as checking input, looping, etc.
The logic should be in the main method in this application.
Wherever you need to modify the household object is where you need to call those setter/getter methods. The constructor is only ever called when you need a brand new object. If this is still confusing, compare the constructor to setting up a game of monopoly. You initially set up the monopoly board, give everyone their money and starting properties, etc. This is all done so that you can actually play the game. When you lose a property in the game, you would use a setter to remove that property. When you need to see how much money, you'd use a getter.
MonopolyPlayer{
int cash;
MonopolyPlayer(){
cash = 1500;
//we're setting this player up to play the game
}
setCash(int i); //now the player has a new amount of cash
getCash(); // he's checking his account
....etc.
}
And so on.