You can create navigation bar programatically this way:
// Create the navigation bar
let navigationBar = UINavigationBar(frame: CGRectMake(0, 20, self.view.frame.size.width, 44)) // Offset by 20 pixels vertically to take the status bar into account
navigationBar.barTintColor = UIColor.whiteColor()
// Create a navigation item with a title
let navigationItem = UINavigationItem()
navigationItem.title = "Profile"
// Assign the navigation item to the navigation bar
navigationBar.items = [navigationItem]
// Make the navigation bar a subview of the current view controller
self.view.addSubview(navigationBar)
Result:
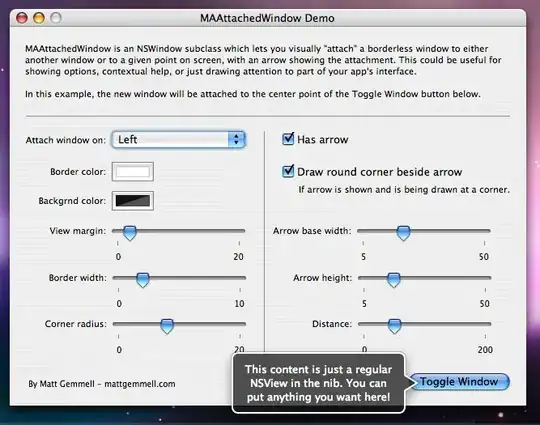
Space is not there anymore.
Original Post: Adding Navigation Bar programmatically iOS
UPDATE:
If you want to add buttons add this code:
// Create left and right button for navigation item
let leftButton = UIBarButtonItem(title: "LeftButton", style: UIBarButtonItemStyle.Plain, target: self, action: "leftClicked:")
let rightButton = UIBarButtonItem(title: "RightButton", style: UIBarButtonItemStyle.Plain, target: self, action: "rightClicked:")
// Create two buttons for the navigation item
navigationItem.leftBarButtonItem = leftButton
navigationItem.rightBarButtonItem = rightButton
And here is helper method:
func leftClicked(sender: UIBarButtonItem) {
// Do something
println("Left Button Clicked")
}
func rightClicked(sender: UIBarButtonItem) {
// Do something
println("Right Button Clicked")
}
And final code will be:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
UIApplication.sharedApplication().statusBarStyle = .LightContent
// Create the navigation bar
let navigationBar = UINavigationBar(frame: CGRectMake(0, 20, self.view.frame.size.width, 44)) // Offset by 20 pixels vertically to take the status bar into account
navigationBar.barTintColor = UIColor.whiteColor()
// Create a navigation item with a title
let navigationItem = UINavigationItem()
navigationItem.title = "Profile"
// Create left and right button for navigation item
let leftButton = UIBarButtonItem(title: "LeftButton", style: UIBarButtonItemStyle.Plain, target: self, action: "leftClicked:")
let rightButton = UIBarButtonItem(title: "RightButton", style: UIBarButtonItemStyle.Plain, target: self, action: "rightClicked:")
// Create two buttons for the navigation item
navigationItem.leftBarButtonItem = leftButton
navigationItem.rightBarButtonItem = rightButton
// Assign the navigation item to the navigation bar
navigationBar.items = [navigationItem]
// Make the navigation bar a subview of the current view controller
self.view.addSubview(navigationBar)
}
func leftClicked(sender: UIBarButtonItem) {
// Do something
println("Left Button Clicked")
}
func rightClicked(sender: UIBarButtonItem) {
// Do something
println("Right Button Clicked")
}
}