The idea behind it is not very difficult but in order to get it to be displayed (at least on ubunto) it gave me some hard time as not all fonts support emoji. I shall display the straight forward way then some links that helped me in the end (maybe you will not need those).
From emoji cheat sheet from the emoji python package I picked up three to be shown as an example and here is the code.
G = nx.Graph()
G.add_nodes_from([0,1,2])
n0 = emoji.emojize(':thumbsup:',use_aliases=True)
n1 = emoji.emojize(':sob:',use_aliases=True)
n2 = emoji.emojize(':joy:',use_aliases=True)
labels ={0:n0,1:n1,2:n2}
nx.draw_networkx(G,labels=labels, node_color = 'w', linewidths=0, with_labels=True, font_family = 'Symbola' ,font_size = 35)
plt.show()
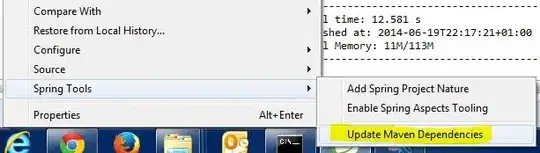
Difficulties encountered:
1- My machine is on ubunto 14.04, I could not display any emoji they always appeared as rectangles
Installed needed font Symbola using the following command (mentioned here):
sudo apt-get install ttf-ancient-fonts
2- Maplotlib (which networkx calls to draw) is not using the installed font.
From several useful discussions 1 2 3 4 5 6 I copied and pasted the .tff font file of Symbola in the default matplotib directory (where it fetches for fonts to use).
cp /usr/share/fonts/truetype/ttf-ancient-scripts/Symbola605.ttf /usr/share/matplotlib/mpl-data/fonts/ttf
Then I had to delete fontList.cache file for the new font to be loaded.
rm ~/.cache/matplotlib/fontList.cache
Note
You can have different views by changing the input to the draw_networkx e.g. not sending the linewidths
will show circular border for each node, also if you want a specific background color for nodes change the color_node
from white to a color that you want ... for more details check the documentation.