You could just google to find a bunch of GagueViews. I think you already did that.
This seems simple. So you could write your own custom View
. You can use drawArc
to draw the 3 different color arc. You can use drawLine
to draw the measuring points (just remember to set Paint.setStrokeCap(Cap.Round)
). As for the needle, you could use drawPath
. With some effort and the right coordinates, you should be able to write a nifty GaugeView yourself.
If you find writing your own view difficult, you can refer some GaugeView
in the GitHub
. You will get a good starting point.
UPDATE: I wrote a simple GaugeView
based on the image in you question. The calculations are in Pixels, you might want to multiply them with DisplayMetrics.density
, so that they are in independent pixels. Also, you might want to expose most of the values here via the xml, so you can control them in the layout. This might be a good starting point.
public class GaugeView extends View {
private Paint arcPaint;
public GaugeView(Context context) {
super(context);
initialize();
}
public GaugeView(Context context, AttributeSet attrs) {
super(context, attrs);
initialize();
}
public GaugeView(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
initialize();
}
private void initialize() {
arcPaint = new Paint(Paint.ANTI_ALIAS_FLAG);
arcPaint.setStyle(Paint.Style.STROKE);
arcPaint.setStrokeWidth(15f);
}
@Override
protected void onDraw(Canvas canvas) {
int width = canvas.getWidth();
int height = canvas.getHeight();
int arcCenterX = width - 10;
int arcCenterY = height - 10;
final RectF arcBounds = new RectF(arcCenterX - 100, arcCenterY - 100, arcCenterX + 100, arcCenterY + 100);
// Draw the arc
canvas.drawArc(arcBounds, 180f, 20f, false, arcPaint);
arcPaint.setColor(Color.DKGRAY);
canvas.drawArc(arcBounds, 200f, 40f, false, arcPaint);
arcPaint.setColor(Color.GRAY);
canvas.drawArc(arcBounds, 2400f, 30f, false, arcPaint);
// Draw the pointers
final int totalNoOfPointers = 20;
final int pointerMaxHeight = 25;
final int pointerMinHeight = 15;
int startX = arcCenterX - 120;
int startY = arcCenterY;
arcPaint.setStrokeWidth(5f);
arcPaint.setStrokeCap(Paint.Cap.ROUND);
int pointerHeight;
for (int i = 0; i <= totalNoOfPointers; i++) {
if(i%5 == 0){
pointerHeight = pointerMaxHeight;
}else{
pointerHeight = pointerMinHeight;
}
canvas.drawLine(startX, startY, startX - pointerHeight, startY, arcPaint);
canvas.rotate(90f/totalNoOfPointers, arcCenterX, arcCenterY);
}
}
}
The final rendered image looks like this:
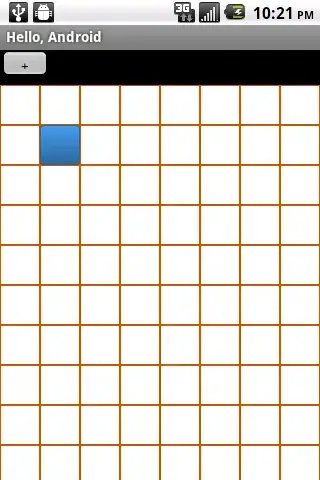