I used ngrok to tunnel the local IP (it is really not that because it is on Google Colab) to a public one.
Going to the ngrok console I can see all the tunnels created. I created just one tunnel for localhost:port but here there are 2, one for HTTP and other for HTTPS (isn't that nice?).

If I go to the https address of my Web App, on the console I see
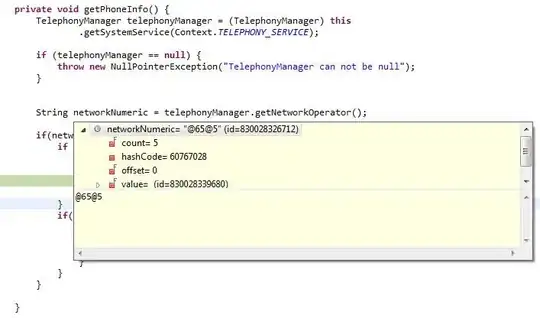
But if I go to the http address, on the console I get
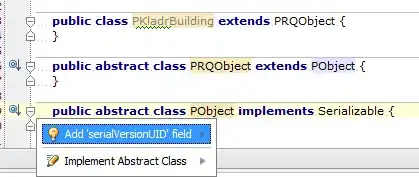
Q: Can you work with service workers that need HTTPs through tunnels to a remote machine?
A: Apparently yes!
The code behind that registration is (important to know where it fails):
// Here we register the SERVICE WORKER
IndexController.prototype._registerServiceWorker = function() {
console.log("1.Starting SW function.");
if (!navigator.serviceWorker) {
console.log("2.Browser is NOT compatible with SW or something is not working.");
return; }
console.log("2.Browser is compatible with SW.");
navigator.serviceWorker.register('/sw.js').then(function() {
console.log('3.Registration worked!');
}).catch(function() {
console.log('3.Registration failed!');
});
};
And to make it more complicated, my web App using Service Workers is running inside Colab (Google Colab). The web App is running on Node.js inside Colab.
If you are working from localhost it should be easier for you, because the https requirement is not enforced when connecting to localhost (according to theory). [A] and [B]

That is not the same that the browser will be nice with your App just because it runs on localhost.
Note: My experiment above..
- Firefox: worked with and without the settings below.
- Chrome: Without adding the urls to the whitelist and restartting I got
Going to https web app I got:
IndexController.js:49 Mixed Content: The page at 'https://0a4e1e0095b0.ngrok.io/' was loaded over HTTPS, but attempted to connect to the insecure WebSocket endpoint 'ws://0a4e1e0095b0.ngrok.io/updates?since=1602934673264&'. This request has been blocked; this endpoint must be available over WSS.
IndexController._openSocket @ IndexController.js:49
IndexController @ IndexController.js:10
(anonymous) @ index.js:16
loadScripts @ loadScripts.js:5
46.../utils/loadScripts @ index.js:15
s @ _prelude.js:1
e @ _prelude.js:1
(anonymous) @ _prelude.js:1
IndexController.js:49 Uncaught DOMException: Failed to construct 'WebSocket': An insecure WebSocket connection may not be initiated from a page loaded over HTTPS.
at IndexController._openSocket (https://0a4e1e0095b0.ngrok.io/js/main.js:2251:12)
Going to http web app I got:
Navigated to http://0a4e1e0095b0.ngrok.io/
IndexController.js:17 1.Starting SW function.
IndexController.js:19 2.Browser is NOT compatible with SW or something is not working.
If you are not on localhost AND can't use https, then you may need to change these settings on your browser.
Some people already explained this but here it goes again.
Chrome:
- Go to chrome://flags/#unsafely-treat-insecure-origin-as-secure
- Add the urls you want to whitelist.
- Restart chrome
Note that this will restart all Chrome windows. This is not a solution for me because my tunnels change name every time they are created and I can't be restarting a bunch of windows every time.
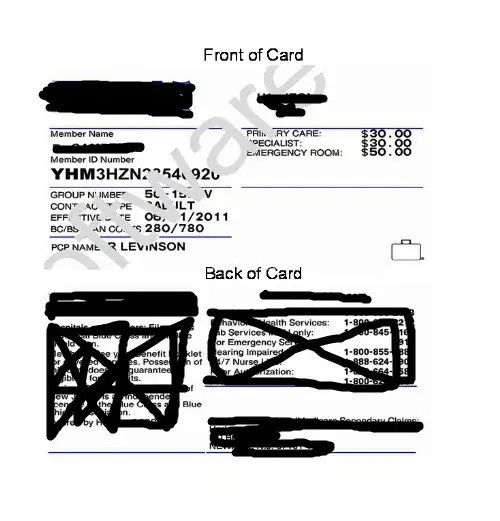
Firefox / Waterfox
- Open Developer Tools
- Open settings
- Mark "Enable service workers over HTTP (when toolbox is open)"
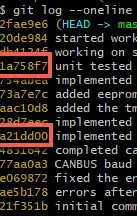
Firefox/Waterfox
You probably don't need to do the changes below, but I did (my browser may be a bit old). More info here.
In about:config
I enabled
dom.serviceWorkers.testing.enabled
dom.serviceWorkers.enabled
I highly recommend looking at this https://developer.mozilla.org/en-US/docs/Web/API/Service_Worker_API/Using_Service_Workers and related pages on that same site.
If someone is interested in the ngrok setup, it is very simple (python version).
# Install pyngrok python package on your Google Colab Session
!pip install pyngrok
# Set up your ngrok Authtoken (requires free registration)
!ngrok authtoken YOUR_TOKEN_HERE
# Invoke ngrok from Python and start tunneling/connecting
from pyngrok import ngrok
# Open a HTTP tunnel on the default port 80 if not specified
ngrok_tunnel = ngrok.connect('8888')
# You can print it, or go to the ngrok console on https://dashboard.ngrok.com/status/tunnels
print (ngrok_tunnel.public_url)