There is a function named imagecopy. This function overrides a part of the destination image using a source image. The given part is specified as parameters. Before you tell me that this does not solve your problem, I have to add that the pixels in the destination picture will not be overriden by the pixels of the source picture if the pixels are transparent. You need to use imagealphablending and imagesavealpha on the source picture, like this:
public static function experimental($images, $width, $height, $dest = null) {
$index = 0;
if ($dest === null) {
$dest = $images[$index++];
}
while ($index < count($images)) {
imagealphablending($images[$index], true);
imagesavealpha($images[$index], true );
imagecopy($dest, $images[$index++], 0, 0, 0, 0, $width, $height);
}
return $dest;
}
If we have these two pictures:
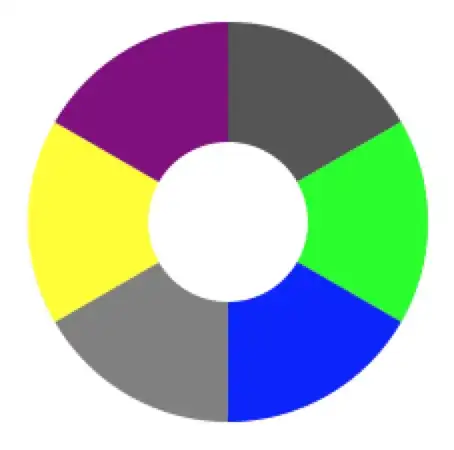
The result will be this:
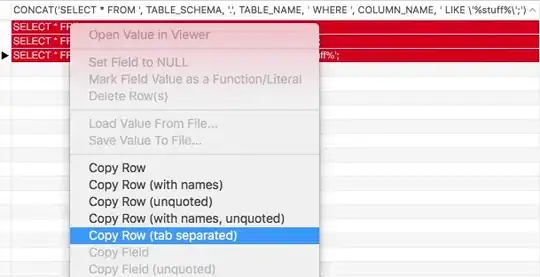