For your original question:
How to add action to a button?
you might want to check How to write an Action Listener.
For your second question:
Now I want to enter another JFrame I made, and make the first disappear. How?
please check both approaches :)
Option 1 (Recommended)
If you want to do it the right way, you should use a CardLayout
as recommended by @AndrewThompson in his comment above.
I also saw you were using a Null Layout (because of setBounds()
method), you might also want to get rid of it, see Why is it frowned upon to use a null layout in Swing? and Null Layout is Evil to know why, insted you should be using a Layout Manager or combinations of them as shown in the following code based on @AndrewThompson's answer (The same that was linked in his comment above) but a bit modified to work with a JFrame
instead of a JOptionPane
, so give him credit by upvoting his Original Answer too!
This produces the following outputs:
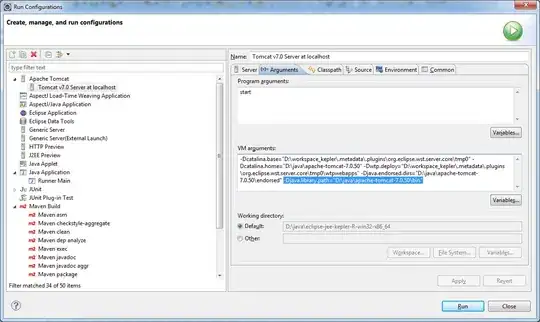
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
class CardLayoutDemo {
JButton button1, button2;
CardLayoutDemo() {
JFrame gui = new JFrame("CardLayoutDemo");
button1 = new JButton("Go to pane 2");
button2 = new JButton("Go to pane 1");
JPanel pane1 = new JPanel();
pane1.setLayout(new BoxLayout(pane1, BoxLayout.PAGE_AXIS));
JPanel pane2 = new JPanel();
pane2.setLayout(new BoxLayout(pane2, BoxLayout.PAGE_AXIS));
final CardLayout cl = new CardLayout();
final JPanel cards = new JPanel(cl);
pane1.add(new JLabel("This is my pane 1"));
pane1.add(button1);
pane2.add(new JLabel("This is my pane 2"));
pane2.add(button2);
gui.add(cards);
cards.add(pane1, "frame1");
cards.add(pane2, "frame2");
ActionListener al = new ActionListener(){
public void actionPerformed(ActionEvent ae) {
if (ae.getSource() == button1) {
cl.show(cards, "frame2");
} else if (ae.getSource() == button2) {
cl.show(cards, "frame1");
}
}
};
button1.addActionListener(al);
button2.addActionListener(al);
gui.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
gui.pack();
gui.setVisible(true);
}
public static void main(String[] args) {
new CardLayoutDemo();
}
}
With this option you only have 1 JFrame
but you can change through different views, and you don't annoy user with multiple windows on the task bar.
One more tip here is: If you're going to open this second JFrame
to prevent user from doing something on the 1st one, you should consider using a JOptionPane
or this second JFrame
will contain just a bit information which you don't want to have there for the whole time (Something like a pop up).
Option 2 (Not recommended)
But if you really really really want to use multiple JFrame
s (which is not recommended) you can dispose()
it. At the time you're calling your new JFrame
to be created. For example, the following code produces this output:
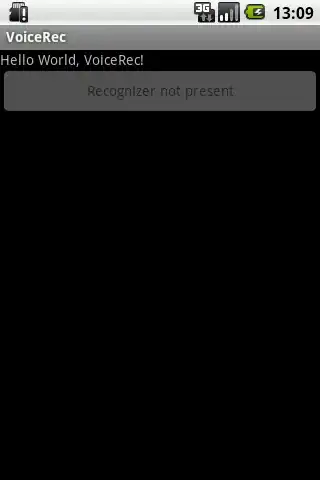
import java.awt.*;
import javax.swing.*;
import java.awt.event.*;
public class TwoJFrames {
JFrame frame;
JButton button;
TwoJFrames() {
frame = new JFrame("1st frame");
button = new JButton("Click me!");
button.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
new AnotherFrame();
frame.dispose();
}
});
frame.add(button);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.pack();
frame.setVisible(true);
}
public static void main(String args[]) {
new TwoJFrames();
}
class AnotherFrame {
JFrame frame2;
JLabel label;
AnotherFrame() {
frame2 = new JFrame("Second Frame");
label = new JLabel("This is my second frame");
frame2.add(label);
frame2.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame2.pack();
frame2.setVisible(true);
}
}
}
In this case you might want to consider setVisible()
instead if you want to go back to previous state or reopen this one when closing the second JFrame
Both of my above codes are called a Minimal, Complete, and Verifiable example (MCVE) or Runnable Example or Short, Self Contained, Correct Example (SSCCE) which are code you can copy-paste and see the same output as me, when you have an error in your code, these examples are very handy because we can see where your errors are or be able to find them easier and/or faster.
You should consider reading all the links I provided (included these ones) and for your future questions to make something like I've done above, that way you'll prevent confusion and you'll get more, faster and better responses.