Wave line with Path
In this approach wavyPath
method will return a Path
object with nested CubicCurbeTo
path elements . Where length
is the length of the line in x axis , subDivisions
are the amount of curves and controlY
is the height in y axis . In this case the method will make a path of 200 pixels length with four curves and 40 pixels amplitude (80 pixels height).
Docs of Path and CubicCurveTo (javafx 19)
This is a single class javafx app you can try
App.java
public class App extends Application {
@Override
public void start(Stage stage) {
var scene = new Scene(new StackPane(wavyPath(200, 4, 40)), 640, 480);
stage.setScene(scene);
stage.show();
}
public static void main(String[] args) {
launch();
}
private Path wavyPath(double length, int subdivisions, int controlY) {
var path = new Path(new MoveTo(0, 0));
double curveLengthX = length / subdivisions;
double controlX = curveLengthX * 0.5;
for (int i = 0; i < subdivisions; i++) {
var curve =
new CubicCurveTo((curveLengthX * i) + controlX, -controlY,
(curveLengthX * i) + controlX, controlY, curveLengthX * (i + 1), 0);
System.out.println(curveLengthX * (1 + i));
path.getElements().add(curve);
}
path.setStroke(Color.PURPLE);
path.setStrokeWidth(3);
return path;
}
}
Result
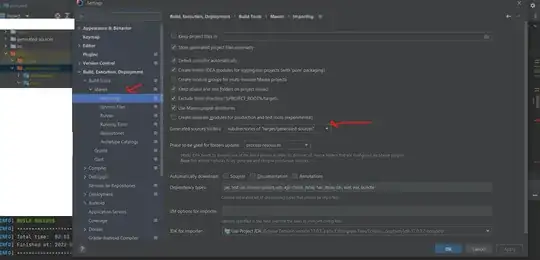