Create Custom Cell XIB file add Progress bar on it
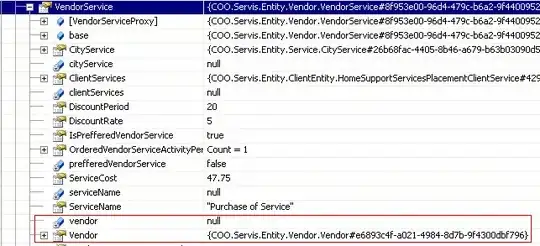
Start download in your view controller, and access progress bar and set progress in didReceiveData method
I just created in view controller, you need to use it with table view methods
Code sample :
@interface ViewController ()<NSURLConnectionDataDelegate>
//Put these in custom cell file
@property (weak, nonatomic) IBOutlet UIProgressView *progressView;
@property (weak, nonatomic) IBOutlet UIImageView *imageView;
@property (strong, nonatomic) NSURLConnection *connectionManager;
@property (strong, nonatomic) NSMutableData *downloadedMutableData;
@property (strong, nonatomic) NSURLResponse *urlResponse;
@end
@implementation ViewController
- (void)viewDidLoad
{
[super viewDidLoad];
//Start download request logic
self.downloadedMutableData = [[NSMutableData alloc] init];
NSURLRequest *urlRequest = [NSURLRequest requestWithURL:[NSURL URLWithString:@"https://www.planwallpaper.com/static/images/63906_1_BdhSun5.jpg"]
cachePolicy:NSURLRequestReloadIgnoringLocalCacheData
timeoutInterval:60.0];
self.connectionManager = [[NSURLConnection alloc] initWithRequest:urlRequest delegate:self];
}
#pragma mark - Delegate Methods
-(void)connection:(NSURLConnection *)connection didReceiveResponse:(NSURLResponse *)response {
NSLog(@"%lld", response.expectedContentLength);
self.urlResponse = response;
}
//Show progress download while receiving data
-(void)connection:(NSURLConnection *)connection didReceiveData:(NSData *)data {
[self.downloadedMutableData appendData:data];
self.progressView.progress = ((100.0/self.urlResponse.expectedContentLength)*self.downloadedMutableData.length)/100;
if (self.progressView.progress == 1) {
self.progressView.hidden = YES;
} else {
self.progressView.hidden = NO;
}
NSLog(@"%.0f%%", ((100.0/self.urlResponse.expectedContentLength)*self.downloadedMutableData.length));
}
-(void)connectionDidFinishLoading:(NSURLConnection *)connection {
NSLog(@"Finished");
self.imageView.image = [UIImage imageWithData:self.downloadedMutableData];
}
Result looks like
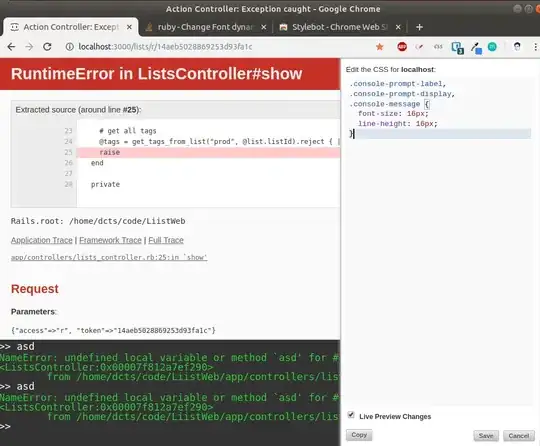