You can use the category for making global setting for UITextField like following:
FOR CREATE CATEGORY check this answer: How to give padding to UITextField in iOS?
UITextField+setBorder.h
#import <UIKit/UIKit.h>
@interface UITextField (setBorder)
-(CGRect)textRectForBounds:(CGRect)bounds;
-(CGRect)editingRectForBounds:(CGRect)bounds;
- (void)setBorderForColor:(UIColor *)color
width:(float)width
radius:(float)radius;
@end
UITextField+setBorder.m
#import "UITextField+setBorder.h"
@implementation UITextField (setBorder)
#pragma clang diagnostic push
#pragma clang diagnostic ignored "-Wobjc-protocol-method-implementation"
-(CGRect)textRectForBounds:(CGRect)bounds {
[self setBorderForColor:[UIColor redColor] width:5 radius:0];
return CGRectMake(bounds.origin.x , bounds.origin.y ,
bounds.size.width , bounds.size.height ); // here you can make coercer position change
}
-(CGRect)editingRectForBounds:(CGRect)bounds {
return [self textRectForBounds:bounds];
}
- (void)setBorderForColor:(UIColor *)color
width:(float)width
radius:(float)radius
{
self.layer.cornerRadius = radius;
self.layer.masksToBounds = YES;
self.layer.borderColor = [color CGColor];
self.layer.borderWidth = width;
}
OUTPUT IS
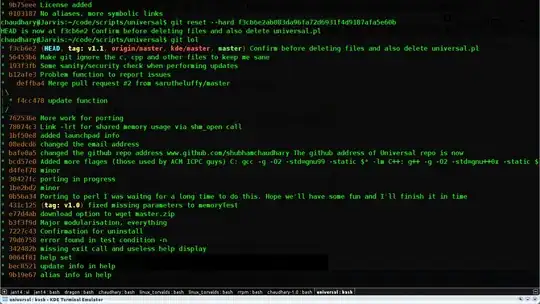