Here is how I've solved this problem:
I've created a form, and override it's OnPaintBackground method like this:
protected override void OnPaintBackground(PaintEventArgs e)
{
using (SolidBrush brush = new SolidBrush(Color.FromArgb(70, 0, 0, 0)))
{
e.Graphics.FillRectangle(brush, e.ClipRectangle);
}
}
Inside this form I'm hosting a user control that's basically a panel with a label and a two buttons (OK, Cancel). Once the user clicks a button I set this form's DialogResult
to OK
or Cancel
(depending on the button clicked).
This form is shown from the main form as a dialog (frm.ShowDialog(this)
).
Also, it takes in it's constructor the main form and set it's own display rectangle to cover the main form completly, and then resize the user control to a third of the height and half of the width of the form, and centers it.
public FrmThickBox(Form owner, string message)
{
this.Owner = owner;
this.Width = owner.Width;
this.Height = owner.Height;
this.Top = owner.Top;
this.Left = owner.Left;
this.thickBoxControl.Text = message;
this.thickBoxControl.Size = new Size((int)this.Width / 2, (int)this.Height / 3);
this.thickBoxControl.Top = (int)((this.Height - thickBoxControl.Height) / 2);
this.thickBoxControl.Left = (int)((this.Width - thickBoxControl.Width) / 2);
}
And here is how it looks like (of course, the rounded corners are an entire different story):
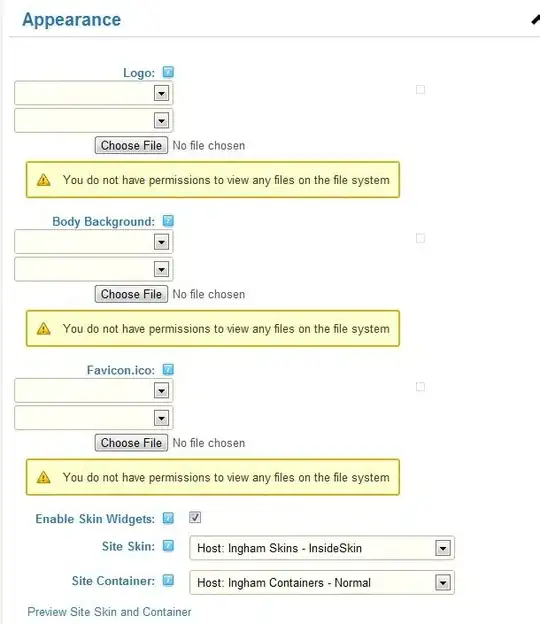