By referring https://stackoverflow.com/a/27505229/72437, I was able to change the background of positive button, without affecting its default margin, padding and selector behavior.
Note that, we don't use colorButtonNormal
, as there is no way to change the button style in Java code.
dimens.xml
<?xml version="1.0" encoding="utf-8"?>
<resources>
<!-- Default insets (outer padding) around buttons -->
<dimen name="button_inset_vertical_material">6dp</dimen>
<dimen name="button_inset_horizontal_material">@dimen/control_inset_material</dimen>
<!-- Default inner padding within buttons -->
<dimen name="button_padding_vertical_material">@dimen/control_padding_material</dimen>
<dimen name="button_padding_horizontal_material">8dp</dimen>
<!-- Default insets (outer padding) around controls -->
<dimen name="control_inset_material">4dp</dimen>
<!-- Default inner padding within controls -->
<dimen name="control_padding_material">4dp</dimen>
<!-- Default rounded corner for controls -->
<dimen name="control_corner_material">2dp</dimen>
</resources>
colors.xml
<color name="rate_app_dialog_positive_button_text_color_material_light">#ffffffff</color>
<color name="rate_app_dialog_positive_button_background_color_material_light">#ff0091ea</color>
<color name="rate_app_dialog_positive_button_pressed_background_color_material_light">#7f0091ea</color>
attrs.xml
<attr name="rateAppDialogPositiveButtonTextColor" format="color" />
<attr name="rateAppDialogPositiveButtonSelector" format="color" />
drawable-v21/rate_app_dialog_positive_button_selector_for_ripple_material_light.xml
<?xml version="1.0" encoding="utf-8"?>
<!-- Used as the canonical button shape. -->
<inset xmlns:android="http://schemas.android.com/apk/res/android"
android:insetLeft="@dimen/button_inset_horizontal_material"
android:insetTop="@dimen/button_inset_vertical_material"
android:insetRight="@dimen/button_inset_horizontal_material"
android:insetBottom="@dimen/button_inset_vertical_material">
<shape android:shape="rectangle"
android:tint="@color/rate_app_dialog_positive_button_background_color_material_light">
<corners android:radius="@dimen/control_corner_material" />
<solid android:color="#ffffffff" />
<padding android:left="@dimen/button_padding_horizontal_material"
android:top="@dimen/button_padding_vertical_material"
android:right="@dimen/button_padding_horizontal_material"
android:bottom="@dimen/button_padding_vertical_material" />
</shape>
</inset>
drawable-v21/rate_app_dialog_positive_button_selector_material_light.xml
<?xml version="1.0" encoding="utf-8"?>
<!-- https://stackoverflow.com/questions/28484369/what-should-be-the-color-of-the-ripple-colorprimary-or-coloraccent-material-d -->
<ripple xmlns:android="http://schemas.android.com/apk/res/android"
android:color="@color/ripple_material_dark">
<!-- @color/ripple_material_light -->
<!-- @color/ripple_material_dark -->
<item android:drawable="@drawable/rate_app_dialog_positive_button_selector_for_ripple_material_light" />
</ripple>
drawable/rate_app_dialog_positive_button_selector_material_light.xml
<?xml version="1.0" encoding="utf-8"?>
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<!-- pressed state -->
<item android:state_pressed="true">
<inset xmlns:android="http://schemas.android.com/apk/res/android"
android:insetLeft="@dimen/button_inset_horizontal_material"
android:insetTop="@dimen/button_inset_vertical_material"
android:insetRight="@dimen/button_inset_horizontal_material"
android:insetBottom="@dimen/button_inset_vertical_material">
<shape android:shape="rectangle">
<corners android:radius="@dimen/control_corner_material" />
<solid android:color="@color/rate_app_dialog_positive_button_pressed_background_color_material_light" />
<padding android:left="@dimen/button_padding_horizontal_material"
android:top="@dimen/button_padding_vertical_material"
android:right="@dimen/button_padding_horizontal_material"
android:bottom="@dimen/button_padding_vertical_material" />
</shape>
</inset>
</item>
<!-- focused state -->
<item android:state_focused="true">
<inset xmlns:android="http://schemas.android.com/apk/res/android"
android:insetLeft="@dimen/button_inset_horizontal_material"
android:insetTop="@dimen/button_inset_vertical_material"
android:insetRight="@dimen/button_inset_horizontal_material"
android:insetBottom="@dimen/button_inset_vertical_material">
<shape android:shape="rectangle">
<corners android:radius="@dimen/control_corner_material" />
<solid android:color="@color/rate_app_dialog_positive_button_pressed_background_color_material_light" />
<padding android:left="@dimen/button_padding_horizontal_material"
android:top="@dimen/button_padding_vertical_material"
android:right="@dimen/button_padding_horizontal_material"
android:bottom="@dimen/button_padding_vertical_material" />
</shape>
</inset>
</item>
<!-- normal state -->
<item>
<inset xmlns:android="http://schemas.android.com/apk/res/android"
android:insetLeft="@dimen/button_inset_horizontal_material"
android:insetTop="@dimen/button_inset_vertical_material"
android:insetRight="@dimen/button_inset_horizontal_material"
android:insetBottom="@dimen/button_inset_vertical_material">
<shape android:shape="rectangle">
<corners android:radius="@dimen/control_corner_material" />
<solid android:color="@color/rate_app_dialog_positive_button_background_color_material_light" />
<padding android:left="@dimen/button_padding_horizontal_material"
android:top="@dimen/button_padding_vertical_material"
android:right="@dimen/button_padding_horizontal_material"
android:bottom="@dimen/button_padding_vertical_material" />
</shape>
</inset>
</item>
</selector>
themes.xml
<item name="rateAppDialogPositiveButtonTextColor">@color/rate_app_dialog_positive_button_text_color_material_light</item>
<item name="rateAppDialogPositiveButtonSelector">@drawable/rate_app_dialog_positive_button_selector_material_light</item>
RateAppDialogFragment.java
TypedValue typedValue = new TypedValue();
Resources.Theme theme = this.getContext().getTheme();
theme.resolveAttribute(R.attr.rateAppDialogPositiveButtonTextColor, typedValue, true);
final int rateAppDialogPositiveButtonTextColor = typedValue.data;
theme.resolveAttribute(R.attr.rateAppDialogPositiveButtonSelector, typedValue, true);
final int rateAppDialogPositiveButtonSelectorResourceId = typedValue.resourceId;
dialog.setOnShowListener(new DialogInterface.OnShowListener() {
@Override
public void onShow(DialogInterface d) {
Button positiveButton = dialog.getButton(AlertDialog.BUTTON_POSITIVE);
positiveButton.setTextColor(rateAppDialogPositiveButtonTextColor);
positiveButton.setBackgroundResource(rateAppDialogPositiveButtonSelectorResourceId);
}
});
This works pretty nice for Android 4 and above.
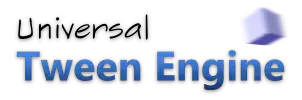