The button widget does not provide this option but you can emulate the button widget to get the effect if required. One way, as mentioned is to use an image containing the rotated text. Depending on your theme you may also create a button using a canvas
which allows you to rotate text drawn on itself using the angle
option. This would look odd on Windows themes but could look normal where the widgets being used are Tk (and not ttk widgets) or with ttk provided the theme is one that uses Tk drawn elements (the default on unix).
A crude demo of how it would look:
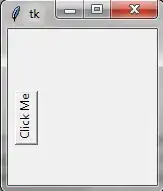
import tkinter as tk
import tkinter.font as tkfont
main = tk.Tk()
font = tkfont.nametofont("TkDefaultFont")
label = "Click Me"
height = font.measure(label) + 4
width = font.metrics()['linespace'] + 4
canvas = tk.Canvas(main, height=height, width=width, background="SystemButtonFace", borderwidth=2, relief="raised")
canvas.create_text((4, 4), angle="90", anchor="ne", text=label, fill="SystemButtonText", font=font)
canvas.bind("<ButtonPress-1>", lambda ev: ev.widget.configure(relief="sunken"))
canvas.bind("<ButtonRelease-1>", lambda ev: ev.widget.configure(relief="raised"))
canvas.place(x=5, y=height + 10)
main.mainloop()