So, I ended up implementing a Firefox Add-on SDK extension that
- Upon Firefox startup: Waits until after the
ready
event fires for the current tab
- After a delay, Opens the Network Monitor
- After an additional delay, Navigates to a webpage (
google.com
in the example code)
- After the ready event fires for that navigation, wait an additional delay and close Firefox.
Currently the page to which to navigate is hard coded. If you need to, it would be possible to make this configurable in a few different ways.
Below is what it looks like when used on Windows 10. jpm run
is from Firefox Add-on SDK development. it allows testing an SDK add-on. You will probably also want to read "jpm run does NOT work with Firefox 48, or later":
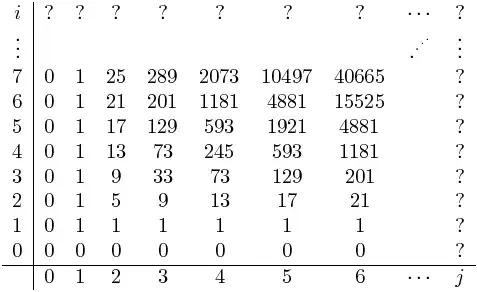
package.json:
{
"title": "Open Network Monitor, navigate, close",
"name": "netmonitor-navigate-close",
"version": "0.0.1",
"description": "Opens the network Monitor, navigates to a page, then closes Firefox",
"main": "index.js",
"author": "Makyen",
"engines": {
"firefox": ">=38.0a1",
"fennec": ">=38.0a1"
},
"license": "MIT",
"keywords": [
"jetpack"
]
}
index.js:
//Opens network monitor, navigates to a page, then closes Firefox.
var pageToNavigateTo = "http://www.google.com";
//Whatever the home page is might have web access happen after
// the ready event. Delay opening the Network monitor so those are skipped.
var delayFirstTabReadyToOpenNetworkmonitor = 3000; //In ms. 3000 = 3 seconds
var delayOpenNetworkmonitorToNavigate = 3000; //In ms. 3000 = 3 seconds
var delayUrlReadyToClose = 5000; //In ms. 5000 = 5 seconds
var tabs = require("sdk/tabs");
var utils = require('sdk/window/utils');
var activeWin = utils.getMostRecentBrowserWindow();
function getActiveWin() {
activeWin = utils.getMostRecentBrowserWindow();
}
getActiveWin();
function openNetworkMonitor(){
activeWin.document.getElementById('menuitem_netmonitor').doCommand();
}
function receiveFirstTabReadyEvent(tab){
getActiveWin();
tabs.off('ready', receiveFirstTabReadyEvent);
activeWin.setTimeout(openNetworkMonitor,delayFirstTabReadyToOpenNetworkmonitor ,tab);
activeWin.setTimeout(navigateToTheUrl,(delayFirstTabReadyToOpenNetworkmonitor
+ delayOpenNetworkmonitorToNavigate) ,tab);
}
function navigateToTheUrl(tab){
tab.on('ready',theUrlIsReady);
tab.url=pageToNavigateTo; //navigate
}
function theUrlIsReady(tab){
tab.off('ready',theUrlIsReady);
getActiveWin();
//Some actions may take place in the page after the ready event. Thus,
// wait some extra time.
activeWin.setTimeout(exitFirefox,delayUrlReadyToClose); //Exit after delay
}
function exitFirefox(){
getActiveWin();
activeWin.document.getElementById('cmd_quitApplication').doCommand();
}
tabs.on('ready', receiveFirstTabReadyEvent);