Maybe SharedPreferences
is what you are looking for
Put this snippet inside any Event handler method e.g. Button
's OnClickListener
:
//creating an instance of a shared preference with code 'edit_text_code' and only reachable by this application
SharedPreferences mySharedPrefs=getSharedPreferences("my_shared_prefs_file_name",MODE_PRIVATE);
//getting the mySharedPrefs's editor for further editing
SharedPreferences.Editor editor=settings.edit();
//putting the EditText content as a shared preference to be 'commited'
editor.putString("edit_text_code",myEditText.getText().toString());
editor.commit();
And now you should read the "shared preference" and its preferences inside, in this case, your EditText
content:
e.g.
public class MainActivity2 extends AppCompatActivity {
TextView myTextView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main2);
myTextView = (TextView) findViewById(R.id.my_text_view);
SharedPreferences mySharedPrefs = getSharedPreferences("my_shared_prefs_file_name",MODE_PRIVATE);
String content = mySharedPrefs.getString("edit_text_code","Show this text in case that shared preference doesn't exist");
textView.setText("The EditText content was: "+ content);
}
For further learning on more topics. Check out this blog:
http://www.vogella.com/tutorials/AndroidFileBasedPersistence/article.html
Try this ChalkSreet app about Android Development also:
https://play.google.com/store/apps/details?id=com.chalkstreet.learnandroid&hl=en
There you can find interesting examples including one very similar to your question
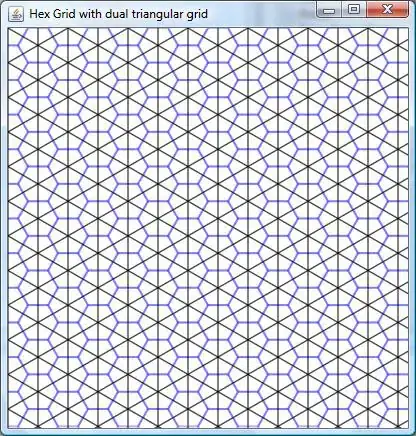

EDIT
Here is the source of the example I was talking about and it Should work.
public class SharedActivity extends AppCompatActivity {
EditText et;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.layout_activity_shared);
et = (EditText) findViewById(R.id.edit_text_shared);
SharedPreferences settings = getSharedPreferences("PREFS",MODE_PRIVATE);
et.setText(settings.getString("option",""));
}
//This does the trick
@Override
protected void onStop() {
super.onStop();
SharedPreferences settings = getSharedPreferences("PREFS",0);
SharedPreferences.Editor editor = settings.edit();
editor.putString("option",et.getText().toString());
editor.commit();
}
}
OR
You can create a file using Java OutputStream
for writing and InputStream
classes to store that "variable"