Given a Series s
you can find the minimum and maximum values like this:
double minX = s.Points.Select(v => v.XValue).Min();
double maxX = s.Points.Select(v => v.XValue).Max();
double minY = s.Points.Select(v => v.YValues[0]).Min();
double maxY = s.Points.Select(v => v.YValues[0]).Max();
Note that they do not directly map into the Points
as each value may occur multiple times. So to find the first and last matching DataPoint
we can use this:
// find datapoints from left..
DataPoint minXpt = s.Points.Select(p => p)
.Where(p => p.XValue == minX)
.DefaultIfEmpty(s.Points.First()).First();
DataPoint minYpt = s.Points.Select(p => p)
.Where(p => p.YValues[0] == minY)
.DefaultIfEmpty(s.Points.First()).First();
//..or from right
DataPoint maxXpt = s.Points.Select(p => p)
.Where(p => p.XValue == maxX)
.DefaultIfEmpty(s.Points.Last()).Last();
DataPoint maxYpt = s.Points.Select(p => p)
.Where(p => p.YValues[0] == maxY)
.DefaultIfEmpty(s.Points.Last()).Last();
Now after marking the found points, maybe like this:
Color c = Color.Green;
minXpt.MarkerColor = c;
minYpt.MarkerColor = c;
maxXpt.MarkerColor = c;
maxYpt.MarkerColor = c;
minXpt.MarkerSize = 12;
minYpt.MarkerSize = 12;
maxXpt.MarkerSize = 12;
maxYpt.MarkerSize = 12;
I get this result:
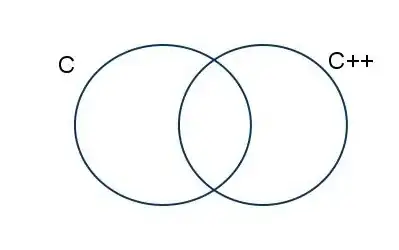
See here for an example how to restrict the search to a zoomed interval!