There are many to consider here, first of them being that your application is an ASP.NET application, and every current user will be different. If your application — just assume — runs correctly on your machine, it will never run on hosting environment because they do not grant write permissions to special folders and user accounts.
That said, you need to work in physical paths in order to create your directories.
var path = "C:\\Users\\afzaa\\Desktop\\";
var folder = Path.Combine(path, "folder");
Directory.CreateDirectory(folder);
The result of the above code is,
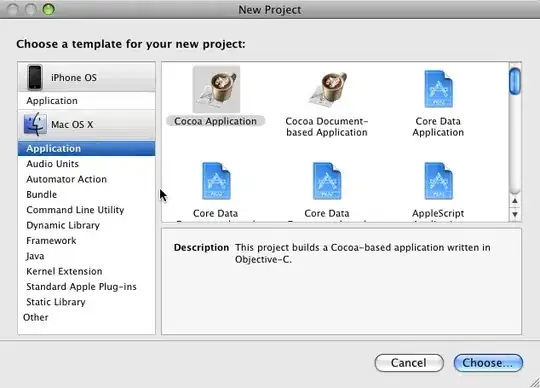
As you can see, the code properly works and has no issue at all in execution.
There are few things to note:
- Your application has read/write permissions. Check IIS for that.
- Your code can actually lookup the path you are trying to access. That applies to any folder inside Desktop too, a sub folder may have special permissions applied.
Do not do this, write the content online in your hosting domain. Users have different accounts and structures for folders and thus this will not work — Desktop path is different.
If you want to users to download the file, simply stream the file down and let them save it where they want to.
https://forums.asp.net/t/1807775.aspx?Create+e+New+Folder+Access+Denied+
https://answers.microsoft.com/en-us/windows/forum/windows_xp-files/unable-to-create-the-folder-new-folder-access-is/ac318218-a7b2-4ee2-b301-2ad91856050b
.NET MVC Access denied when trying to create Directory