Unless you want to decrease your plotting area (i.e. not plot some points), you can still have "full" control over your y limits while using scales = "free_y"
.
You can use the same trick I have given to answer your other question: how to set limits on rounded facet wrap y axis?
dat <- data.table(dat)
dat[,y_min := y*0.5, by = group]
dat[,y_max:= y*1.5, by = group]
ggplot(dat, aes(x = x, y = y)) +
geom_point() +
geom_line() +
facet_wrap(~group, ncol = 1, scales = "free_y") +
geom_blank(aes(y = y_min)) +
geom_blank(aes(y = y_max))
For others reading this question, trick is to explicitly create y_min
and y_max
variables for each group. And "plot" them via geom_blank()
. (Nothing is actually plotted, but each facet's plotting area is adjusted based on y_min
and y_max
values for that group).
If for some reasons, you want to manually give min and max (instead of a rule), none is stopping you. But it is tedious:
dat[group == "a",y_min := 0]
dat[group == "a",y_max := 30]
dat[group == "b",y_min := 0]
dat[group == "b",y_max := 3000]
ggplot(dat, aes(x = x, y = y)) +
geom_point() +
geom_line() +
facet_wrap(~group, ncol = 1, scales = "free_y") +
geom_blank(aes(y = y_min)) +
geom_blank(aes(y = y_max))
But, as I have mentioned this works if you want to extend your limits, not decrease them.
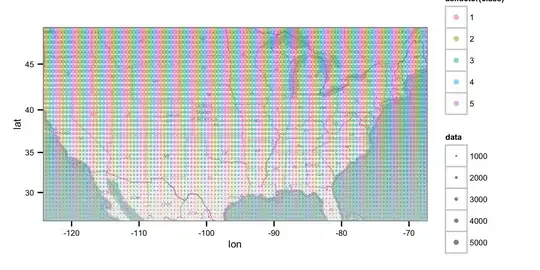