Combined your code with the code for onMeasure from the answer I mentioned in comments:
public class CrashView extends View {
private int width, height, bheight, bwidth, boxwidth, boxheight;
private float x, y, vx, vy;
private Bitmap bullet;
private Paint AA = new Paint(Paint.ANTI_ALIAS_FLAG);
public CrashView(Context context) {
super(context);
init(context);
}
public CrashView(Context context, @Nullable AttributeSet attrs) {
super(context, attrs);
init(context);
}
public CrashView(Context context, @Nullable AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init(context);
}
@TargetApi(Build.VERSION_CODES.LOLLIPOP)
public CrashView(Context context, @Nullable AttributeSet attrs, int defStyleAttr, int defStyleRes) {
super(context, attrs, defStyleAttr, defStyleRes);
init(context);
}
private void init(Context ctx) {
Display display = ((Activity) ctx).getWindowManager().getDefaultDisplay();
Point size = new Point();
display.getSize(size);
//REPLACE DRAWABLE HERE
bullet = BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher);
bullet = Bitmap.createScaledBitmap(bullet, bullet.getWidth() / 5, bullet.getHeight() / 5, true);
width = size.x;
height = size.y;
bheight = bullet.getHeight();
bwidth = bullet.getWidth();
boxheight = height / 4;
boxwidth = (width / 20) * 19;
x = (width / 20) / 4;
y = boxheight - bheight / 4 * 3;
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
int desiredWidth = boxwidth;
int desiredHeight = boxwidth;
int widthMode = MeasureSpec.getMode(widthMeasureSpec);
int widthSize = MeasureSpec.getSize(widthMeasureSpec);
int heightMode = MeasureSpec.getMode(heightMeasureSpec);
int heightSize = MeasureSpec.getSize(heightMeasureSpec);
int width;
int height;
//Measure Width
if (widthMode == MeasureSpec.EXACTLY) {
//Must be this size
width = widthSize;
} else if (widthMode == MeasureSpec.AT_MOST) {
//Can't be bigger than...
width = Math.min(desiredWidth, widthSize);
} else {
//Be whatever you want
width = desiredWidth;
}
//Measure Height
if (heightMode == MeasureSpec.EXACTLY) {
//Must be this size
height = heightSize;
} else if (heightMode == MeasureSpec.AT_MOST) {
//Can't be bigger than...
height = Math.min(desiredHeight, heightSize);
} else {
//Be whatever you want
height = desiredHeight;
}
//MUST CALL THIS
setMeasuredDimension(width, height);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
vy = -0.1f - (float) ((Math.pow(x / 600, 2)) / 1.25);
x += vx;
y += vy;
canvas.drawBitmap(bullet, x, y, AA);
invalidate();
}
}
The XMl is as simple as:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/root">
<com.test.myapplication.CrashView
android:layout_width="wrap_content"
android:layout_height="wrap_content"/>
</RelativeLayout>
Here is the layout with displaying bounds on:
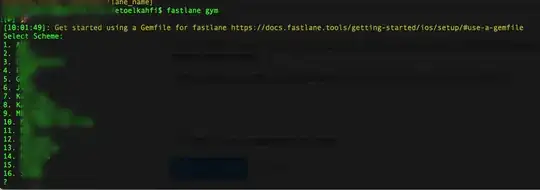
Things to keep in mind:
- Magic numbers - you got quite a few of those here, you know best what are they for but it's better to keep them as named constants
- View size depends on size of a screen. This might not be a good idea. It should depend on the size of the resource you are drawing.
- I used relative layout but it should be no different with constraint layout.
- Extra constructors that appeared were needed for XML inflation