If you are using the FXyz library you can very easily apply different textures to an icosahedron or to any of the different primitives you can find in the library.
This snippet shows 5 different texture modes:
@Override
public void start(Stage primaryStage) {
PerspectiveCamera camera = new PerspectiveCamera(true);
camera.setTranslateY(3);
camera.setTranslateX(4);
camera.setTranslateZ(-15);
IcosahedronMesh icoLine = new IcosahedronMesh(100, 0);
icoLine.setDrawMode(DrawMode.LINE);
icoLine.getTransforms().addAll(new Rotate(10, Rotate.X_AXIS), new Rotate(-20, Rotate.Y_AXIS));
IcosahedronMesh icoColor = new IcosahedronMesh(100, 0);
icoColor.setTextureModeNone(Color.LIGHTGREEN);
icoColor.getTransforms().addAll(new Rotate(20, Rotate.X_AXIS), new Rotate(-20, Rotate.Y_AXIS));
IcosahedronMesh icoFunction = new IcosahedronMesh(100, 0);
icoFunction.setTextureModeVertices3D(1530, p -> Math.cos(p.z));
icoFunction.getTransforms().addAll(new Rotate(30, Rotate.X_AXIS), new Rotate(-20, Rotate.Y_AXIS));
IcosahedronMesh icoFaces = new IcosahedronMesh(100, 0);
icoFaces.setTextureModeFaces(5);
icoFaces.getTransforms().addAll(new Rotate(20, Rotate.X_AXIS), new Rotate(-10, Rotate.Y_AXIS));
IcosahedronMesh icoImage = new IcosahedronMesh(100, 0);
icoImage.setTextureModeImage(getClass().getResource("icon.jpg").toExternalForm());
icoImage.getTransforms().addAll(new Rotate(20, Rotate.X_AXIS), new Rotate(-20, Rotate.Y_AXIS));
IcosahedronMesh icoPattern = new IcosahedronMesh(100, 0);
icoPattern.setTextureModePattern(Patterns.CarbonPatterns.CARBON_KEVLAR, 100);
icoPattern.getTransforms().addAll(new Rotate(20, Rotate.X_AXIS), new Rotate(-30, Rotate.Y_AXIS));
GridPane grid = new GridPane();
grid.add(new Group(icoLine), 0, 0);
grid.add(new Group(icoColor), 1, 0);
grid.add(new Group(icoFunction), 2, 0);
grid.add(new Group(icoFaces), 0, 1);
grid.add(new Group(icoImage), 1, 1);
grid.add(new Group(icoPattern), 2, 1);
Scene scene = new Scene(grid, 600, 400, true, SceneAntialiasing.BALANCED);
scene.setCamera(camera);
primaryStage.setScene(scene);
primaryStage.setTitle(("Icosahedron - FXyz3D"));
primaryStage.show();
}
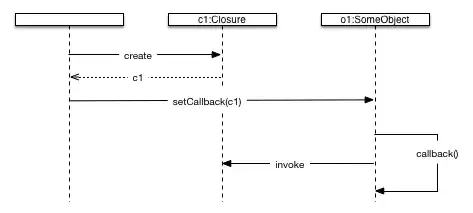