When you import a 3D model with a third-party 3D importer you have less control of the resulting TriangleMesh. If you want to provide texture features to your model you'll have to edit the exported file and add the texture coordinates, which is not the best approach.
But if you could generate the mesh from scratch, you could easily apply textures over it.
This question shows how you can define the texture coordinates and uses the same net image you have to provide the texture of an icosahedron.
Based on the answer on that question, the texture can be defined without an actual image, just with a palette of colors.
And you can easily change those on runtime, i.e. when you click on one face you can change the color on that face.
The Fxyz library makes use of a TexturedMesh, designed to easily apply textures to 3D shapes.
You can find many primitives there, like the icosahedron.
This question shows the result of different texture modes over an icosahedron.
This short snippet shows how you can apply a texture over faces, and change it on runtime:
private int numColors = 10;
@Override
public void start(Stage primaryStage) {
PerspectiveCamera camera = new PerspectiveCamera(true);
camera.setTranslateZ(-5);
IcosahedronMesh icoFaces = new IcosahedronMesh(100, 0);
icoFaces.setTextureModeFaces(numColors);
icoFaces.getTransforms().addAll(new Rotate(20, Rotate.X_AXIS), new Rotate(-10, Rotate.Y_AXIS));
final Group group = new Group(icoFaces);
Scene scene = new Scene(group, 600, 400, true, SceneAntialiasing.BALANCED);
scene.setCamera(camera);
primaryStage.setScene(scene);
primaryStage.setTitle(("Icosahedron - FXyz3D"));
primaryStage.show();
icoFaces.setOnMouseClicked(e -> {
ObservableFaceArray faces = ((TriangleMesh) icoFaces.getMesh()).getFaces();
int selectedFace = e.getPickResult().getIntersectedFace();
int colorId = faces.get(6 * selectedFace + 1);
int newColorId = colorId + 1 >= numColors ? 0 : colorId + 1;
faces.set(6 * selectedFace + 1, newColorId);
faces.set(6 * selectedFace + 3, newColorId);
faces.set(6 * selectedFace + 5, newColorId);
});
}
Running the application:

And after clicking in the frontal green face:
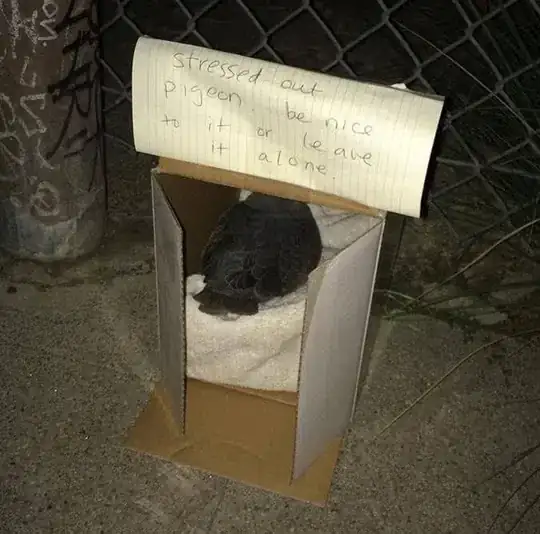