Best way to show Text
on button
(with image)
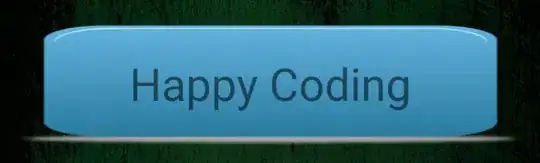
Your Question: How to show text
on imagebutton
?
Answer: You can not display text
with imageButton
. Method that tell in Accepted answer also not work.
because
If you use android:drawableLeft="@drawable/buttonok"
then you can not set drawable
in center
of button
.
If you use android:background="@drawable/button_bg"
then color
of your drawable
will be changed.
In android world there are thousands of option to do this. But here i provide best alternate according to my point of view. (see below)
Solution: Use cardView
with LinearLayout
Your drawable/image
use in LinearLayout
because it shows in center. And with help of textView
you can set text
on this. We makes cardView
background to transparent
.
<androidx.cardview.widget.CardView
android:layout_width="match_parent"
android:layout_height="99dp"
android:layout_margin="16dp"
app:cardBackgroundColor="@android:color/transparent"
app:cardElevation="0dp"
app:cardUseCompatPadding="true">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/your_selected_image"
>
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="Happy Coding"
android:textSize="33sp"
android:gravity="center"
>
</TextView>
</LinearLayout>
</androidx.cardview.widget.CardView>
here i explain some terms:
app:cardBackgroundColor="@android:color/transparent"
for make transparent background of cardView
app:cardElevation="0dp"
for hide evelation lines around cardView
app:cardUseCompatPadding="true"
its provide actual size of cardView
. Always use this when you use cardView
set your image/drawable
in LinearLayout
as a background.
Sorry, for my Bad English.
Happy Coding:)