Let start point is P0, end point P1, angle Fi. R is not needed
At first find arc center. Get middle of P0-P1 segment.
M = (P0 + P1) / 2
// M.x = (P0.x + P1.x) / 2 , same for y
And direction vector
D = (P1 - P0) / 2
Get length of D
lenD = Math.Hypot(D.x, D.y) //Vector.Length, sqrt of sum of squares
Get unit vector
uD = D / lenD
Get (left) perpendicular vector
(P.x, P.y) = (-uD.y, ud.x)
Now circle center
if F = Pi then
C.x = M.x
C.y = M.y
else
C.x = M.x + P.x * Len / Tan(Fi/2)
C.y = M.y + P.y * Len / Tan(Fi/2)
Vector from center to start point:
CP0.x = P0.x - C.x
CP0.y = P0.y - C.y
Then you can calculate coordinates of N intermediate points at the arc using rotation of vector CP0 around center point
an = i * Fi / (NSeg + 1);
X[i] = C.x + CP0.x * Cos(an) - CP0.y * Sin(an)
Y[i] = C.y + CP0.x * Sin(an) + CP0.y * Cos(an)
Working Delphi code
procedure ArcByStartEndAngle(P0, P1: TPoint; Angle: Double; NSeg: Integer);
var
i: Integer;
len, dx, dy, mx, my, px, py, t, cx, cy, p0x, p0y, an: Double;
xx, yy: Integer;
begin
mx := (P0.x + P1.x) / 2;
my := (P0.y + P1.y) / 2;
dx := (P1.x - P0.x) / 2;
dy := (P1.y - P0.y) / 2;
len := Math.Hypot(dx, dy);
px := -dy / len;
py := dx / len;
if Angle = Pi then
t := 0
else
t := len / Math.Tan(Angle / 2);
cx := mx + px * t;
cy := my + py * t;
p0x := P0.x - cx;
p0y := P0.y - cy;
for i := 0 to NSeg + 1 do begin
an := i * Angle / (NSeg + 1);
xx := Round(cx + p0x * Cos(an) - p0y * Sin(an));
yy := Round(cy + p0x * Sin(an) + p0y * Cos(an));
Canvas.Ellipse(xx - 3, yy - 3, xx + 4, yy + 4);
end;
end;
Result for (Point(100, 0), Point(0, 100), Pi / 2, 8
(Y-axis down at the picture)
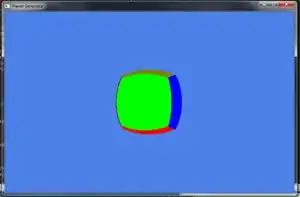