Well I had seen a same error few days back I stumbled upon something called eval function, that is how you can perhaps try this running,
private isUScustomer: Boolean;
this.myservice.isUSCustomer()
.subscribe(x => {
this.isUScustomer = eval(x);
});
Edit 1
TS look for return type and datatype for http calls, you can perhaps try to create an interface and give this a go. Let me show you how,
an Interface like this,
export interface booleanReturn{
retData: boolean;
}
Import this in your service and use it as given below,
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { HttpBackend } from '@angular/common/http/src/backend';
import { booleanReturn } from '../interfaces/MyInterfaces';
import { Observable } from 'rxjs/Observable';
@Injectable()
export class MyServiceService {
constructor(private httpClient: HttpClient) { }
getBoolean(): Observable<booleanReturn>{
return this.httpClient.get<booleanReturn>('http://localhost:8080/getBoolean');
}
}
Now in your component do something like this,
import { Component } from '@angular/core';
import { MyServiceService } from './my-service.service';
import { booleanReturn } from '../interfaces/MyInterfaces';
/*interface booleanReturn{
retData: boolean
}*/
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'app';
myObj ={"decision":'2==2'};
private myBooleanVal : booleanReturn;
constructor(private myService:MyServiceService){
this.getBooleanValue();
}
myFunction(vwLog){
//console.log(eval(vwLog),"Dj test");
return eval(vwLog);
}
getBooleanValue(){
this.myService.getBoolean().subscribe(x=>{
this.myBooleanVal = x;
console.log(x,"X Value");// this print true "X Value"
console.log(this.myBooleanVal);// this prints "true"
});
}
}
see my screenshot below I can see the correct answer
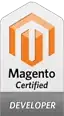