I have used InputDecoration.collapsed to remove the divider and I am changing the color of the bottom border.
If you enter a name the bottom border color is blue and if you enter a number or other special characters then the bottom border color is red
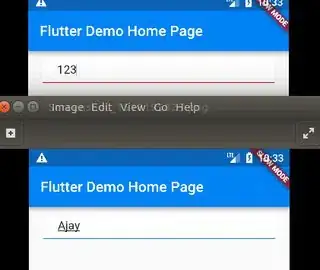
import 'package:flutter/material.dart';
void main() => runApp(new MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return new MaterialApp(
title: 'Flutter Demo',
home: new MyHomePage(title: 'Flutter Demo Home Page'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => new _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
static const EdgeInsets _padding = const EdgeInsets.symmetric(horizontal: 20.0, vertical: 8.0);
Color borderColor = Colors.blue;
bool nameFlag = false;
@override
void initState() {
super.initState();
}
void validateName(String value) {
final RegExp nameExp = new RegExp(r'^[A-Za-z ]+$');
if (!nameExp.hasMatch(value) || value.isEmpty)
borderColor = Colors.red;
else
borderColor = Colors.blue;
}
@override
Widget build(BuildContext context) {
return new Scaffold(
appBar: new AppBar(
title: new Text(widget.title),
),
body: new Column(children: <Widget>[
new Flexible(
child: new Container(
margin: _padding,
padding: _padding,
child: new TextField(
decoration: new InputDecoration.collapsed(
hintText: "Enter Name",
),
onChanged: (s) {
setState(() => validateName(s));
},
),
decoration: new BoxDecoration(
color: Colors.white,
border: new Border(
bottom: new BorderSide(color: borderColor, style: BorderStyle.solid),
),
),
),
)
]),
);
}
}
Let me know if this answers your question :)