Option 1:
if you are looking for something like facebook,Linkedin you just need one variable isOpen. If isOpen true return MoreText else LessText (use substring) .
May not be a perfect solution but you can get what you need
Warning!!! you have to handle text length when using substring
import 'package:flutter/material.dart';
import 'package:linky/presentation/extension/utils.dart';
class ExpandableText extends StatefulWidget {
final String text;
final double max;
const ExpandableText({Key? key, required this.text, required this.max})
: super(key: key);
@override
_ExpandableTextState createState() => _ExpandableTextState();
}
class _ExpandableTextState extends State<ExpandableText> {
TextPainter? textPainter;
bool isOpen = false;
@override
Widget build(BuildContext context) {
return isOpen
? SizedBox(
child: Align(
alignment: Alignment.centerLeft,
child: RichText(
textAlign: TextAlign.start,
text: TextSpan(children: [
TextSpan(
text: widget.text, style: textTheme(context).bodyText1),
WidgetSpan(
child: InkWell(
onTap: () {
setState(() {
isOpen = !isOpen;
});
},
child: Text(
"Less more",
style: textTheme(context).bodyText1!.copyWith(
fontWeight: FontWeight.bold,
color: Colors.blueAccent),
)),
style: textTheme(context).bodyText1)
]),
)))
: Align(
alignment: Alignment.centerLeft,
child: RichText(
textAlign: TextAlign.start,
maxLines: 2,
text: TextSpan(children: [
TextSpan(
text: widget.text.substring(
0,
int.parse(
"${(widget.text.length * widget.max).toInt()}")) +
"...",
style: textTheme(context).bodyText1),
WidgetSpan(
child: InkWell(
mouseCursor: SystemMouseCursors.click,
onTap: () {
setState(() {
isOpen = !isOpen;
});
},
child: Text(
"more",
style: textTheme(context).bodyText1!.copyWith(
fontWeight: FontWeight.bold,
color: Colors.blueAccent),
)),
style: textTheme(context).bodyText1)
]),
),
);
}
}
Exemple :
ExpandableText(
text: users[0].loremIpsum,
max: 0.2,
)
Option 2: If you need something like ExpansionPanel you juste use widget ExpansionTile .
ExpansionTile(title: title,children: [])
Inside Childress moreText & title your LessText . You can hide LessText when isOpen
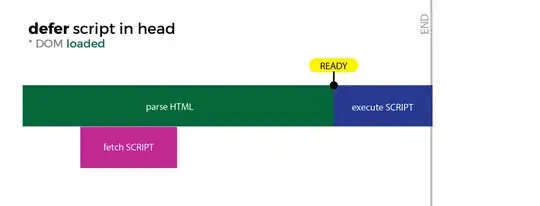