You will first need to generate a grid of x
and y
points at which to evaluate your function f(x,y)
. You can do this using meshgrid
. For example, this will create 201-by-201 point grids from -1 to 1 for both x
and y
:
[x, y] = meshgrid(-1:0.01:1);
Now you can fill a matrix z
with the results of f(x,y)
, evaluating each part at different subsets of grid points using logical indexing:
z = 5.*x+4.*y; % Use g(x,y) as default fill value
index = ((x-y) < -0.5); % Logical index of grid points for h(x,y)
z(index) = x(index).^2+y(index).^2; % Evaluate h(x,y) at index points
index = ((x-y) >= 0); % Logical index of grid points for k(x,y)
z(index) = x(index).*y(index); % Evaluate k(x,y) at index points
Now you can plot z
using surf
:
surf(x, y, z, 'EdgeColor', 'none'); % Plots surface without edge lines
Then you can generate corner coordinates for the planes and plot them with patch
(see this question linked in a comment from bla for more details). Here's one way to generate them (making them red with some alpha transparency):
xPlane = [-1 1 1 -1]; % X coordinates of plane corners, ordered around the plane
yPlane1 = xPlane+0.5; % Corresponding y coordinates for plane 1
yPlane2 = xPlane; % Corresponding y coordinates for plane 2
zPlane = [-10 -10 10 10]; % Z coordinates of plane corners
hold on; % Add to existing plot
patch(xPlane, yPlane1, zPlane, 'r', 'FaceAlpha', 0.5); % Plot plane 1
patch(xPlane, yPlane2, zPlane, 'r', 'FaceAlpha', 0.5); % Plot plane 2
And here's the resulting plot:
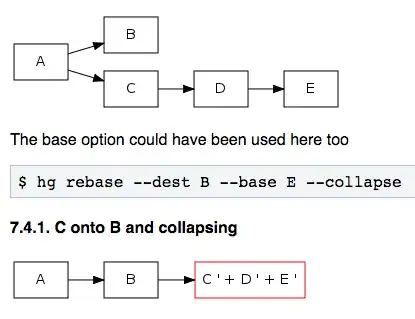