About the comments:
The use of Thread.Sleep()
is just an example to test the results of a local instance of the Random class when used in a close loop.
You will, probably, get series of the same result. Or all identical values.
Here, as a test, I'm inserting a small pause (1ms
) when returning the random color, so the colors will be selected using a different seed (almost) every time. Test it without the pause.
The default implementation, using a single instance of the Random class, can yield similar results.
private Color[] Colors = { Color.Red, Color.Yellow, Color.Green};
private Color GetColor()
{
Random random = new Random(DateTime.Now.Millisecond);
Thread.Sleep(1);
return Colors[random.Next(0,3)];
}
Test it without the Thread.Sleep()
pause:
for (int i = 0; i < 100; i++)
{
Console.WriteLine(GetColor().Name);
}
Or, more properly, using a static Field:
private static Random random = new Random();
private Color GetColor()
{
return Colors[random.Next(0,3)];
}
A slightly different method to get different shades of Red
, Green
and Yellow
for each element:
(It should probably be tweaked a little to avoid semi-gray color).
private Color GetColor2()
{
Color color = Colors[random.Next(0, 3)];
switch (color.Name)
{
case "Yellow":
color = Color.FromArgb((160 + random.Next(0, 96)), (160 + random.Next(0, 96)), 0);
break;
case "Red":
color = Color.FromArgb((160 + random.Next(0, 96)), 0, 0);
break;
case "Green":
color = Color.FromArgb(0, (160 + random.Next(0, 96)), 0);
break;
}
return color;
}
This is a random palette this method generates:
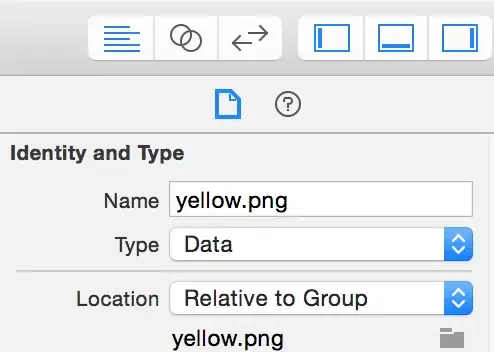