Like @hichame has said, your button is not getting bigger. You can read this and this to understand why your button looks like bigger.
About the button's color, you can refer to this use android.R.drawable.btn_default
:
button.SetBackgroundResource(Android.Resource.Drawable.ButtonDefault);
to reset your button. But, the result is not like the origin button.
Finally, I get the default button's color :#D6D7D7
.
You can use this value to reset your button's color to default:
button.Background.SetTintList(ColorStateList.ValueOf(Color.ParseColor("#D6D7D7")));
Update:
After read this answer, I find a solution, you need use AppCompatButton
, install Xamarin.Android.Support.v7.AppCompat, use it in your layout:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<android.support.v7.widget.AppCompatButton
android:id="@+id/bt1"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
<android.support.v7.widget.AppCompatButton
android:id="@+id/bt2"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
Use SupportBackgroundTintList
in your MainActivity
( also that is why your return value is null):
public class MainActivity : AppCompatActivity,View.IOnClickListener
{
AppCompatButton bt1;
ColorStateList backgroundTintList;
public void OnClick(View v)
{
bt1.SupportBackgroundTintList=(backgroundTintList);
//bt1.SetBackgroundResource(Android.Resource.Drawable.ButtonDefault);
}
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
// Set our view from the "main" layout resource
SetContentView(Resource.Layout.activity_main);
bt1 = FindViewById<AppCompatButton>(Resource.Id.bt1);
AppCompatButton bt2 = FindViewById<AppCompatButton>(Resource.Id.bt2);
backgroundTintList = bt2.SupportBackgroundTintList;
bt1.SupportBackgroundTintList=ColorStateList.ValueOf(Color.Red);
bt2.SetOnClickListener(this);
}
}
Result:
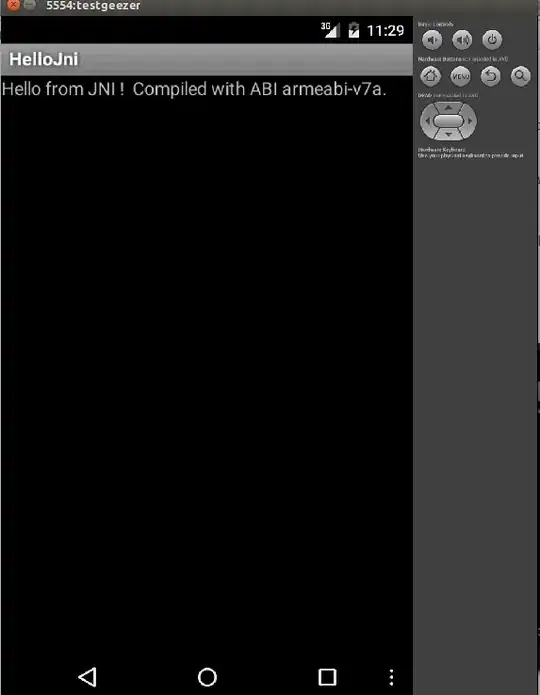