There are several possible approaches. For example, a pure vector-based approach could be used. However, the conversion of the individual drawings is likely to be quite time-consuming.
A more pragmatic approach could be as follows:
Approach
You could define a list of Bézier paths (UIBezierPath), each describing a region that can be drawn independently.
The Bézier paths would serve two purposes:
- determine in which area a stroke has begun
- limit the user drawing to this area
How to draw perfectly inside the black lines
If you make the background of the given drawing transparent, you can create the user's drawing operations with clipping below the black line drawing. This means that the Bézier path does not have to be perfect, it just has to be sufficiently accurate.
Layers/Clipping
The steps would be then:
- show initially the given drawing
- determine the Bézier path where the user starts drawing in
- clip to determined Bézier path
- draw user strokes with clipping
- draw on top the given transparaent line drawing
Quick Demo
Here is a short demo to show what it might look like when the user starts drawing within a clipped Bézier path using the described drawing order (see also code snippets below):
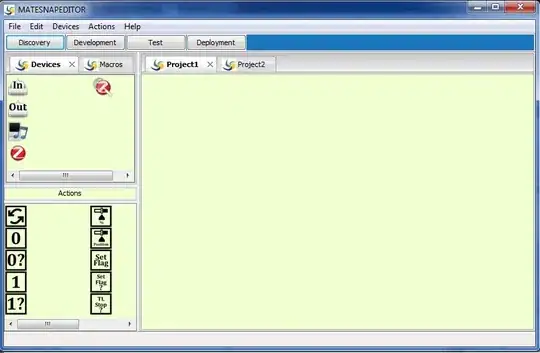
Code Snippets
Example bezier path
bezierPath = UIBezierPath()
bezierPath.move(to: CGPoint(x: 55.94, y: 60.19))
bezierPath.addCurve(to: CGPoint(x: 50.32, y: 94.09), controlPoint1: CGPoint(x: 56.92, y: 59.69), controlPoint2: CGPoint(x: 55.25, y: 73.99))
bezierPath.addCurve(to: CGPoint(x: 48.81, y: 115.93), controlPoint1: CGPoint(x: 46.9, y: 107.97), controlPoint2: CGPoint(x: 49.09, y: 115.33))
bezierPath.addCurve(to: CGPoint(x: 74.36, y: 146.45), controlPoint1: CGPoint(x: 50.56, y: 127.4), controlPoint2: CGPoint(x: 60.85, y: 141.06))
bezierPath.addCurve(to: CGPoint(x: 119.11, y: 126.29), controlPoint1: CGPoint(x: 93.52, y: 141.34), controlPoint2: CGPoint(x: 107.61, y: 134.31))
bezierPath.addCurve(to: CGPoint(x: 223.27, y: 93.88), controlPoint1: CGPoint(x: 150.33, y: 112.49), controlPoint2: CGPoint(x: 183.59, y: 100.44))
bezierPath.addCurve(to: CGPoint(x: 300.41, y: 69.01), controlPoint1: CGPoint(x: 250.68, y: 87.71), controlPoint2: CGPoint(x: 276.22, y: 79.2))
bezierPath.addCurve(to: CGPoint(x: 297.23, y: 59.69), controlPoint1: CGPoint(x: 301.38, y: 65.82), controlPoint2: CGPoint(x: 300.22, y: 62.01))
bezierPath.addCurve(to: CGPoint(x: 212.85, y: 38.3), controlPoint1: CGPoint(x: 290.2, y: 62.67), controlPoint2: CGPoint(x: 256.59, y: 52.37))
bezierPath.addCurve(to: CGPoint(x: 137.05, y: 37.2), controlPoint1: CGPoint(x: 191.43, y: 31.36), controlPoint2: CGPoint(x: 158.26, y: 29.92))
bezierPath.addCurve(to: CGPoint(x: 100.74, y: 48.08), controlPoint1: CGPoint(x: 126.18, y: 40.93), controlPoint2: CGPoint(x: 111.78, y: 44.75))
bezierPath.addCurve(to: CGPoint(x: 55.94, y: 60.19), controlPoint1: CGPoint(x: 86.15, y: 52.48), controlPoint2: CGPoint(x: 70.56, y: 56.69))
bezierPath.close()
Clip to path
self.currentBezierPath.addClip()
Determining if a touch start inside a closed bezier path
Assuming that self is a custom view, one could write something like:
override func touchesBegan(_ touches: Set<UITouch>, with event: UIEvent?) {
...
let point = touch.location(in: self)
...
let inside = bezierPath.contains(point)
...
Draw transparaent drawing
First one has to make the background of the drawing transparent. Then one could store the drawing in a format that supports transparaency, e.g. .png.
context.saveGState()
context.scaleBy(x: 1, y: -1)
context.translateBy(x: 0, y: -oOV7Impng.size.height)
context.draw(oOV7Impng.cgImage!, in: CGRect(x: 0, y: 0, width: oOV7Impng.size.width, height: oOV7Impng.size.height))
context.restoreGState()
If there are many drawings in the app, one could think about making the background of the image programmatically transparent (e.g. by making white pixels transparent). Depends on the use case.